diff options
author | Mark Stosberg <mark@rideamigos.com> | 2023-03-10 02:19:20 -0500 |
---|---|---|
committer | GitHub <noreply@github.com> | 2023-03-09 23:19:20 -0800 |
commit | eed3eb0b1da0c9499ad26d5d4042644a39b7b576 (patch) | |
tree | ae11f8e9d691a88a65a29682e754dc7039b8f5a0 /keyboards | |
parent | 313fc4cb8f4e41c921112d0b105b82ad5d8a653e (diff) |
[Keymap] add crkbd/keymaps/markstos (#19010)
Co-authored-by: Drashna Jaelre <drashna@live.com>
Co-authored-by: Ryan <fauxpark@gmail.com>
Diffstat (limited to 'keyboards')
-rw-r--r-- | keyboards/crkbd/keymaps/markstos/config.h | 62 | ||||
-rw-r--r-- | keyboards/crkbd/keymaps/markstos/keymap.c | 112 | ||||
-rw-r--r-- | keyboards/crkbd/keymaps/markstos/readme.md | 15 | ||||
-rw-r--r-- | keyboards/crkbd/keymaps/markstos/rules.mk | 11 |
4 files changed, 200 insertions, 0 deletions
diff --git a/keyboards/crkbd/keymaps/markstos/config.h b/keyboards/crkbd/keymaps/markstos/config.h new file mode 100644 index 0000000000..ff00a04a8d --- /dev/null +++ b/keyboards/crkbd/keymaps/markstos/config.h @@ -0,0 +1,62 @@ +/* +This is the C configuration file for the keymap + + Copyright 2022 Mark Stosberg (@markstos) + SPDX-License-Identifier: GPL-2.0-or-later + +*/ + +#pragma once + +//#define USE_MATRIX_I2C + +/* Select hand configuration */ + +// #define MASTER_LEFT +#define MASTER_RIGHT +// #define EE_HANDS + +//#define SSD1306OLED + + +// By default, when holding a dual-function key shortly after tapping it, the +// tapped key will begin repeating. This is handy for fast typists when typing +// words with double letters, such as "happy". If you turn this setting ON, it +// will be counted as a held modifier instead. +//#define TAPPING_FORCE_HOLD + +// Customized by markstos +#define TAPPING_TERM 200 +#define TAPPING_TERM_PER_KEY +// used for Tapping Term on thumb keys +#define TAPPING_TERM_THUMB 125 + +// If you press a dual-role key, press another key, and then release the +// dual-role key, all within the tapping term, by default the dual-role key +// will perform its tap action. If the HOLD_ON_OTHER_KEY_PRESS option is +// enabled, the dual-role key will perform its hold action instead. +#define HOLD_ON_OTHER_KEY_PRESS + +// markstos: not sure if these are correct +// They are intended to beep and flash during flashing +#define QMK_LED D5 +#define QMK_SPEAKER C6 + +// Prevent normal rollover on alphas from accidentally triggering mods. +#define IGNORE_MOD_TAP_INTERRUPT + +// When enabled, typing a mod-tap plus second within term will register as the mod-combo +// Ref: https://beta.docs.qmk.fm/using-qmk/software-features/tap_hold#permissive-hold +#define PERMISSIVE_HOLD + +#define COMBO_COUNT 2 + +// Set the COMBO_TERM so low that I won't type the keys one after each other during normal typing. +// They would have be held together intentionally to trigger this. +#define COMBO_TERM 40 + +// These mostly affect my one-shot Shift key, providing a CapsLock alternative. +// I want a relatively low timeout, so if I accidentally type "Shift", I can pause just briefly and move on. +#define ONESHOT_TAP_TOGGLE 3 /* Tapping this number of times holds the key until tapped once again. */ +#define ONESHOT_TIMEOUT 2000 /* Time (in ms) before the one shot key is released */ + diff --git a/keyboards/crkbd/keymaps/markstos/keymap.c b/keyboards/crkbd/keymaps/markstos/keymap.c new file mode 100644 index 0000000000..ca5be183b1 --- /dev/null +++ b/keyboards/crkbd/keymaps/markstos/keymap.c @@ -0,0 +1,112 @@ +// Copyright 2022 Mark Stosberg (@markstos) +// SPDX-License-Identifier: GPL-2.0-or-later +#include QMK_KEYBOARD_H + +enum custom_keycodes { + QWERTY = SAFE_RANGE, + LOWER, + RAISE, + FUNC, + BACKLIT +}; + +enum combos { + DF_DASH, + JK_ESC +}; + +const uint16_t PROGMEM df_combo[] = {KC_D, KC_F, COMBO_END}; +const uint16_t PROGMEM jk_combo[] = {KC_J, KC_K, COMBO_END}; + +combo_t key_combos[COMBO_COUNT] = { + // Add commonly used dash to home row + [DF_DASH] = COMBO(df_combo, KC_MINS), + // For Vim, put Escape on the home row + [JK_ESC] = COMBO(jk_combo, KC_ESC), +}; + +// Each layer gets a name for readability, which is then used in the keymap matrix below. +// The underscores don't mean anything - you can have a layer called STUFF or any other name. +// Layer names don't all need to be of the same length, obviously, and you can also skip them +// entirely and just use numbers. +enum custom_layers { + _QWERTY, + _LOWER, + _RAISE, + _FUNC, +}; + +// For _QWERTY layer +#define OSM_LCTL OSM(MOD_LCTL) +#define OSM_AGR OSM(MOD_RALT) +#define OSL_FUN OSL(_FUNC) +#define GUI_ENT GUI_T(KC_ENT) +#define LOW_TAB LT(_LOWER, KC_TAB) +#define RSE_BSP LT(_RAISE, KC_BSPC) +#define OSM_SFT OSM(MOD_LSFT) + + +// For _RAISE layer +#define CTL_ESC LCTL_T(KC_ESC) + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + [_QWERTY] = LAYOUT( + //,-----------------------------------------------------. ,-----------------------------------------------------. + KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y ,KC_U ,KC_I ,KC_O ,KC_P ,KC_DEL , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + OSM(MOD_LALT), KC_A, KC_S, KC_D, KC_F, KC_G, KC_H ,KC_J ,KC_K ,KC_L ,KC_QUOT ,OSM_AGR , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + OSM(MOD_LSFT), KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N ,KC_M ,KC_COMM ,KC_DOT ,KC_SLSH ,OSL_FUN , + //|--------+--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------+--------| + OSM_LCTL, GUI_ENT, LOW_TAB, RSE_BSP ,KC_SPC ,OSM_SFT + //`--------------------------' `--------------------------' + ), + + [_LOWER] = LAYOUT( + //,-----------------------------------------------------. ,-----------------------------------------------------. + _______, KC_EXLM, KC_AT, KC_HASH, KC_DLR, KC_PERC, KC_CIRC, KC_AMPR, KC_ASTR, KC_LPRN, KC_RPRN, _______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, _______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, XXXXXXX , KC_TILD,KC_GRV, KC_LBRC, KC_LCBR, KC_RCBR, KC_RBRC, KC_COMM,KC_DOT, KC_SLSH, _______ , + //|--------+--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------+--------| + KC_TRNS, KC_TRNS, LOWER, KC_TRNS, KC_TRNS, KC_COLON + //`--------------------------' `--------------------------' + ), + + + [_RAISE] = LAYOUT( + //,-----------------------------------------------------. ,-----------------------------------------------------. + _______, KC_DEL , XXXXXXX, KC_UNDS, KC_PLUS, KC_PGUP, XXXXXXX, XXXXXXX, XXXXXXX, KC_BSLS, KC_PIPE,_______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, KC_HOME, KC_END , KC_MINS, KC_EQL , KC_PGDN, KC_LEFT, KC_DOWN, KC_UP , KC_RGHT, KC_APP ,_______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, KC_LT , KC_GT , KC_COPY, KC_PSTE, KC_SCLN, KC_MPLY, KC_MPRV, KC_MNXT, KC_VOLD, KC_VOLU,_______ , + //|--------+--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------+--------| + CTL_ESC, KC_TRNS, XXXXXXX, RAISE , KC_TRNS, KC_TRNS + //`--------------------------' `--------------------------' + ), + + [_FUNC] = LAYOUT( + //,-----------------------------------------------------. ,-----------------------------------------------------. + _______, KC_F1 , KC_F2 , KC_F3 , KC_F4 , KC_F5 , KC_F6 , KC_F7 , KC_F8 , KC_F9 , KC_F10 ,_______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, KC_F11 , KC_F12 , XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX , XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,_______ , + //|--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------| + _______, KC_CAPS, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX , XXXXXXX, XXXXXXX, XXXXXXX, QK_BOOT,XXXXXXX , + //|--------+--------+--------+--------+--------+--------+--------| |--------+--------+--------+--------+--------+--------+--------| + XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, FUNC , XXXXXXX + //`--------------------------' `--------------------------' + ) +}; + +uint16_t get_tapping_term(uint16_t keycode, keyrecord_t *record) { + switch (keycode) { + case LT(_RAISE, KC_BSPC): + return TAPPING_TERM_THUMB; + case LT(_LOWER, KC_TAB): + return TAPPING_TERM_THUMB; + default: + return TAPPING_TERM; + } +} diff --git a/keyboards/crkbd/keymaps/markstos/readme.md b/keyboards/crkbd/keymaps/markstos/readme.md new file mode 100644 index 0000000000..6789c9da30 --- /dev/null +++ b/keyboards/crkbd/keymaps/markstos/readme.md @@ -0,0 +1,15 @@ +# Markstos Corne keyboard layout + +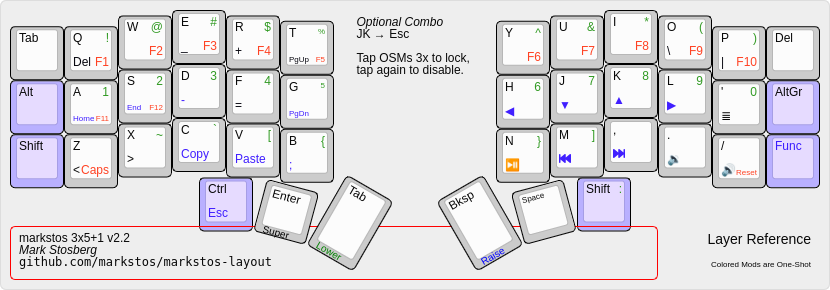 + +A primarily 3x5 layout for split ergonomic keywords with an extra column on each hand for rare and optional keys. + +For a detailed description see [markstos Corne layout](https://mark.stosberg.com/markstos-corne-3x5-1-keyboard-layout). + +# Disclaimer + +This is my personal layout and is subject to evolve further with my tastes. Fork your own copy if you need stability. Suggestions welcome. + +# Author + +* [Mark Stosberg](mailto:mark@stosberg.com) diff --git a/keyboards/crkbd/keymaps/markstos/rules.mk b/keyboards/crkbd/keymaps/markstos/rules.mk new file mode 100644 index 0000000000..9bca23db95 --- /dev/null +++ b/keyboards/crkbd/keymaps/markstos/rules.mk @@ -0,0 +1,11 @@ +# markstos: enable media keys +EXTRAKEY_ENABLE = yes + +# markstos: smaller file size, little down-side +LTO_ENABLE = yes + +COMBO_ENABLE = yes + +# This is for RGB *underglow* +# https://github.com/qmk/qmk_firmware/blob/master/docs/feature_rgblight.md +RGBLIGHT_ENABLE = no |