diff options
author | QMK Bot <hello@qmk.fm> | 2021-07-03 15:14:07 +0000 |
---|---|---|
committer | QMK Bot <hello@qmk.fm> | 2021-07-03 15:14:07 +0000 |
commit | c82e0d64a2ba74af04c3e0d739ab6ef6bc246f26 (patch) | |
tree | 4c943bf767f0591d2b8583830cbd7de1bb77cc6f | |
parent | 19daf3da23549af5249d6a4cd37118ab3ffdaff9 (diff) | |
parent | 002d90b3577dc09416ef1491b65f65bbf5438b01 (diff) |
Merge remote-tracking branch 'origin/master' into develop
-rwxr-xr-x | keyboards/mechwild/mercutio/keymaps/jonavin/keymap.c | 148 | ||||
-rw-r--r-- | keyboards/mechwild/mercutio/keymaps/jonavin/readme.md | 24 | ||||
-rw-r--r-- | keyboards/mechwild/mercutio/keymaps/jonavin/rules.mk | 1 |
3 files changed, 155 insertions, 18 deletions
diff --git a/keyboards/mechwild/mercutio/keymaps/jonavin/keymap.c b/keyboards/mechwild/mercutio/keymaps/jonavin/keymap.c index f5dddcfac8..6fadea4899 100755 --- a/keyboards/mechwild/mercutio/keymaps/jonavin/keymap.c +++ b/keyboards/mechwild/mercutio/keymaps/jonavin/keymap.c @@ -26,19 +26,38 @@ enum custom_layers { _RAISE, }; +enum custom_keycodes { + ENCFUNC = SAFE_RANGE, // encoder function keys +}; + +// Tap Dance Definitions +enum custom_tapdance { + TD_LSFT_CAPSLOCK, +}; + +qk_tap_dance_action_t tap_dance_actions[] = { + // Tap once for shift, twice for Caps Lock + [TD_LSFT_CAPSLOCK] = ACTION_TAP_DANCE_DOUBLE(KC_LSFT, KC_CAPS), +}; + +#define KC_LSFTCAPS TD(TD_LSFT_CAPSLOCK) +#define KC_CAD LALT(LCTL(KC_DEL)) +#define KC_AF4 LALT(KC_F4) +#define KC_TASK LCTL(LSFT(KC_ESC)) + const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { [_BASE] = LAYOUT_all( KC_MUTE, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_BSPC, TT(_RAISE), KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, - KC_LSFT, KC_SLSH, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SFTENT, + KC_LSFTCAPS, KC_SLSH, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SFTENT, KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, LT(_LOWER,KC_SPC), KC_SPC, KC_RALT, MO(_FN1), KC_RCTL ), [_FN1] = LAYOUT_all( - KC_TRNS, + ENCFUNC, KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_DEL, - KC_CAPS, KC_F11, KC_F12, KC_NO, KC_NO, KC_NO, KC_NO, KC_PSCR, KC_SCLN, KC_PAUS, KC_NO, KC_NO, - KC_TRNS, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NLCK, KC_P0, KC_NO, KC_NO, KC_SFTENT, + KC_CAPS, KC_F11, KC_F12, KC_NO, KC_NO, KC_NO, KC_NO, KC_PSCR, KC_SLCK, KC_PAUS, KC_NO, KC_NO, + KC_TRNS, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NLCK, KC_NO, KC_NO, KC_NO, KC_SFTENT, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS ), [_LOWER] = LAYOUT_all( @@ -56,8 +75,64 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { KC_TRNS, KC_TRNS, KC_TRNS, KC_BSPC, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS ) }; +/* These are needed whether encoder function is enabled or not when ENCFUNC keycode is pressed. + Defaults never changes if no encoder present to change it +*/ +typedef struct { + char keydesc[6]; // this will be displayed on OLED + uint16_t keycode; // this is the keycode that will be sent when activted +} keycodedescType; + +static const keycodedescType PROGMEM keyselection[] = { + // list of key codes that will be scrollled through by encoder and description + {"TASK", KC_TASK}, + {"INS", KC_INS}, + {"DEL", KC_DEL}, + {"PrtSc", KC_PSCR}, + {"ScrLk", KC_SCLN}, + {"Break", KC_PAUS}, + {"C-A-D", KC_CAD}, // Ctrl-Alt-Del + {"AltF4", KC_AF4}, + {"PLAY", KC_MEDIA_PLAY_PAUSE} +}; + +#define MAX_KEYSELECTION sizeof(keyselection)/sizeof(keyselection[0]) + +static uint8_t selectedkey_idx = 0; +static keycodedescType selectedkey_rec; + +static void set_selectedkey(uint8_t idx) { + // make a copy from PROGMEM + memcpy_P (&selectedkey_rec, &keyselection[idx], sizeof selectedkey_rec); + + //selectedkey_rec = keyselection[idx]; + +} + +void keyboard_post_init_user(void) { + // Call the keyboard post init code. + //selectedkey_rec = keyselection[selectedkey_idx]; + set_selectedkey(selectedkey_idx); +} + +bool process_record_user(uint16_t keycode, keyrecord_t *record) { + switch (keycode) { + case ENCFUNC: + if (record->event.pressed) { + tap_code16(selectedkey_rec.keycode); + } else { + // when keycode is released + } + break; + } + return true; +}; + + + #ifdef ENCODER_ENABLE // Encoder Functionality uint8_t selected_layer = 0; + bool encoder_update_user(uint8_t index, bool clockwise) { #ifdef OLED_DRIVER_ENABLE oled_clear(); @@ -65,21 +140,54 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { #endif switch (index) { case 0: // This is the only encoder right now, keeping for consistency - if ( clockwise ) { - if ( selected_layer < 3 && keyboard_report->mods & MOD_BIT(KC_LSFT) ) { // If you are holding L shift, encoder changes layers - selected_layer ++; - layer_move(selected_layer); + switch(get_highest_layer(layer_state)){ // special handling per layer + case _FN1: // on Fn layer select what the encoder does when pressed + if (!keyboard_report->mods) { + if ( clockwise ) { + if ( selectedkey_idx < MAX_KEYSELECTION-1) { + selectedkey_idx ++; + } else { + // do nothing + } + } else if ( !clockwise ) { + if ( selectedkey_idx > 0){ + selectedkey_idx --; + } else { + // do nothing + } + } + set_selectedkey(selectedkey_idx); + break; } else { - tap_code(KC_VOLU); // Otherwise it just changes volume + // continue to default } - } else if ( !clockwise ) { - if ( selected_layer > 0 && keyboard_report->mods & MOD_BIT(KC_LSFT) ){ - selected_layer --; - layer_move(selected_layer); - } else { - tap_code(KC_VOLD); + default: // all other layers + if ( clockwise ) { + if ( selected_layer < 3 && keyboard_report->mods & MOD_BIT(KC_LSFT) ) { // If you are holding L shift, encoder changes layers + selected_layer ++; + layer_move(selected_layer); + } else if (keyboard_report->mods & MOD_BIT(KC_LCTL)) { // if holding Left Ctrl, navigate next word + tap_code16(LCTL(KC_RGHT)); + } else if (keyboard_report->mods & MOD_BIT(KC_LALT)) { // if holding Left Alt, change media next track + tap_code(KC_MEDIA_NEXT_TRACK); + } else { + tap_code(KC_VOLU); // Otherwise it just changes volume + } + } else if ( !clockwise ) { + if ( selected_layer > 0 && keyboard_report->mods & MOD_BIT(KC_LSFT) ) { + selected_layer --; + layer_move(selected_layer); + } else if (keyboard_report->mods & MOD_BIT(KC_LCTL)) { // if holding Left Ctrl, navigate previous word + tap_code16(LCTL(KC_LEFT)); + } else if (keyboard_report->mods & MOD_BIT(KC_LALT)) { // if holding Left Alt, change media previous track + tap_code(KC_MEDIA_PREV_TRACK); + } else { + tap_code(KC_VOLD); + } } + break; } + break; } return true; } @@ -129,12 +237,15 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { } render_logo(); oled_set_cursor(8,2); + char fn_str[12]; switch(selected_layer){ case 0: oled_write_P(PSTR("BASE"), false); break; case 1: - oled_write_P(PSTR("FN"), false); + sprintf(fn_str, "FN %5s", selectedkey_rec.keydesc); + oled_write(fn_str, false); + //oled_write_P(PSTR("FN "), false); break; case 2: oled_write_P(PSTR("LOWER"), false); @@ -147,14 +258,15 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { } oled_set_cursor(8,3); if (get_highest_layer(layer_state) == selected_layer) { - oled_write_P(PSTR(" "), false); + oled_write_P(PSTR(" "), false); } else { switch (get_highest_layer(layer_state)) { case 0: oled_write_P(PSTR("Temp BASE"), false); break; case 1: - oled_write_P(PSTR("Temp FN"), false); + sprintf(fn_str, "Temp FN %5s", selectedkey_rec.keydesc); + oled_write(fn_str, false); break; case 2: oled_write_P(PSTR("Temp LOWER"), false); diff --git a/keyboards/mechwild/mercutio/keymaps/jonavin/readme.md b/keyboards/mechwild/mercutio/keymaps/jonavin/readme.md index 5122f143d5..9180ac215c 100644 --- a/keyboards/mechwild/mercutio/keymaps/jonavin/readme.md +++ b/keyboards/mechwild/mercutio/keymaps/jonavin/readme.md @@ -12,5 +12,29 @@ Features - MOUSEKEYS is disbled - shutdown oled when powered down to prevent OLED from showing Mercutio all the time - add WPM indicator when wpm is > 20 wpm + - add double tap of Left Shift to toggle Caps Lock + + - FN layer has encoder selectable key codes and displayed on OLED + + Change these in keymap.c to assign your desired key selection + static const keycodedescType PROGMEM keyselection[] = { + // list of key codes that will be scrollled through by encoder and description + {"TASK", KC_TASK}, + {"INS", KC_INS}, + {"DEL", KC_DEL}, + {"PrtSc", KC_PSCR}, + {"ScrLk", KC_SCLN}, + {"Break", KC_PAUS}, + {"C-A-D", KC_CAD}, // Ctrl-Alt-Del + {"AltF4", KC_AF4}, + {"PLAY", KC_MEDIA_PLAY_PAUSE} + }; + + - Additional encoder functionality + While holding Left Ctrl, navigates next or previous word + While holding Left Alt, media next track or previous track + + +Default Layers 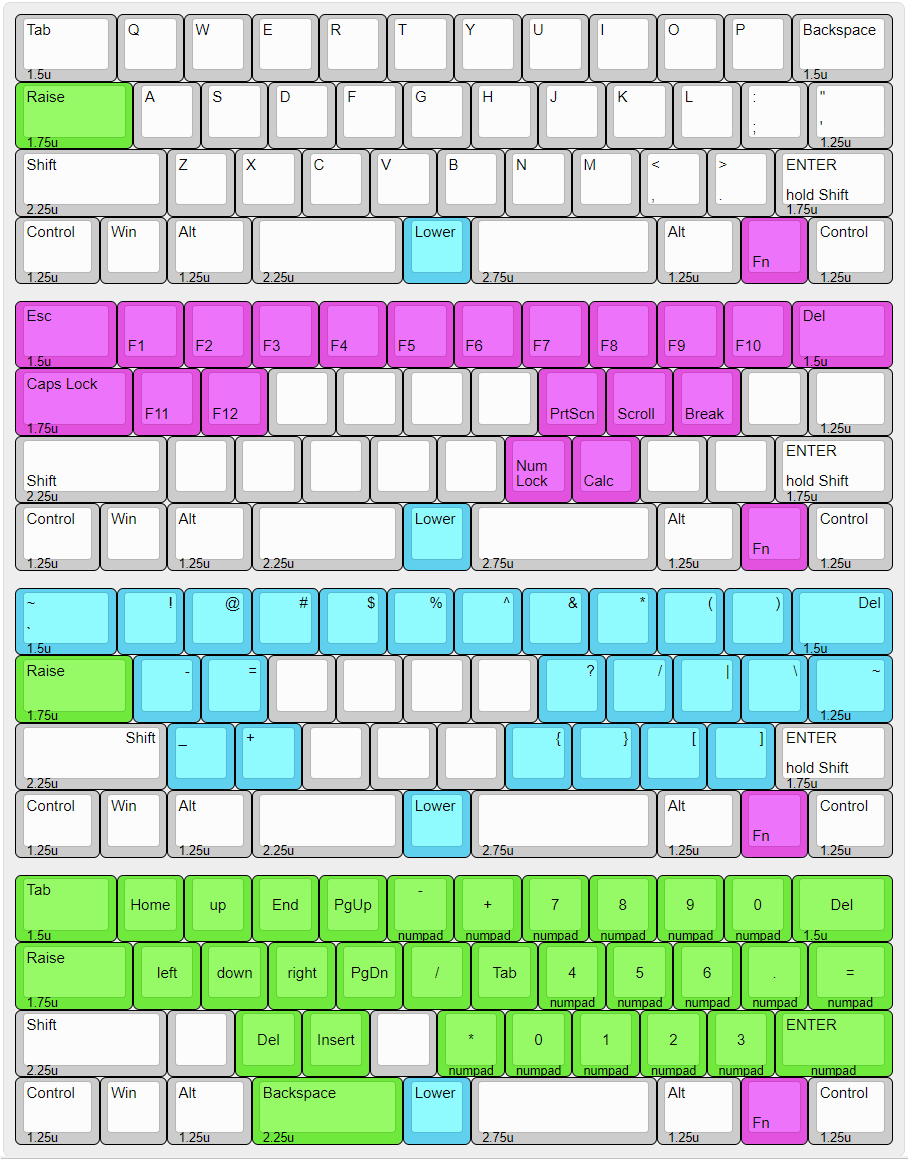 diff --git a/keyboards/mechwild/mercutio/keymaps/jonavin/rules.mk b/keyboards/mechwild/mercutio/keymaps/jonavin/rules.mk index 5ae6c7d311..550e84c809 100644 --- a/keyboards/mechwild/mercutio/keymaps/jonavin/rules.mk +++ b/keyboards/mechwild/mercutio/keymaps/jonavin/rules.mk @@ -2,3 +2,4 @@ VIA_ENABLE = yes MOUSEKEY_ENABLE = yes WPM_ENABLE = yes +TAP_DANCE_ENABLE = yes |