diff options
Diffstat (limited to 'keyboards')
79 files changed, 2035 insertions, 216 deletions
diff --git a/keyboards/duck/duck_led/duck_led.c b/keyboards/duck/duck_led/duck_led.c new file mode 100644 index 0000000000..2fa920e4b6 --- /dev/null +++ b/keyboards/duck/duck_led/duck_led.c @@ -0,0 +1,7 @@ +#include <avr/io.h> +#include "duck_led.h" +#include "quantum.h" + +void show(void) { + wait_us((RES / 1000UL) + 1); +} diff --git a/keyboards/duck/duck_led/duck_led.h b/keyboards/duck/duck_led/duck_led.h new file mode 100644 index 0000000000..2546366d0c --- /dev/null +++ b/keyboards/duck/duck_led/duck_led.h @@ -0,0 +1,16 @@ +#pragma once + +#define RES 6000 + +#define NS_PER_SEC (1000000000L) +#define CYCLES_PER_SEC (F_CPU) +#define NS_PER_CYCLE (NS_PER_SEC / CYCLES_PER_SEC) +#define NS_TO_CYCLES(n) ((n) / NS_PER_CYCLE) + +enum Device { + Device_PCBRGB, + Device_STATUSLED +}; + +void show(void); + diff --git a/keyboards/duck/eagle_viper/readme.md b/keyboards/duck/eagle_viper/readme.md index 14fb166698..3fec11bc0a 100644 --- a/keyboards/duck/eagle_viper/readme.md +++ b/keyboards/duck/eagle_viper/readme.md @@ -9,4 +9,3 @@ Newest version is the [Eagle/Viper V2](http://duck0113.tistory.com/127) Make example for this keyboard (after setting up your build environment): make duck/eagle_viper/v2:default - diff --git a/keyboards/duck/eagle_viper/v2/config.h b/keyboards/duck/eagle_viper/v2/config.h index f454b2e0e1..a0ce866cc3 100644 --- a/keyboards/duck/eagle_viper/v2/config.h +++ b/keyboards/duck/eagle_viper/v2/config.h @@ -15,8 +15,7 @@ You should have received a copy of the GNU General Public License along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef CONFIG_H -#define CONFIG_H +#pragma once #include "config_common.h" @@ -44,6 +43,9 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_DI_PIN D6 #define RGBLED_NUM 17 +/* Set to top left most key */ +#define BOOTMAGIC_LITE_ROW 4 +#define BOOTMAGIC_LITE_COLUMN 10 + #define TAPPING_TERM 200 -#endif diff --git a/keyboards/duck/eagle_viper/v2/indicator_leds.c b/keyboards/duck/eagle_viper/v2/indicator_leds.c index 03a93197da..fc90ed3fbd 100644 --- a/keyboards/duck/eagle_viper/v2/indicator_leds.c +++ b/keyboards/duck/eagle_viper/v2/indicator_leds.c @@ -19,19 +19,13 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #include <stdbool.h> #include <util/delay.h> #include "indicator_leds.h" - -#define RES 6000 +#include "duck_led/duck_led.h" #define LED_T1H 600 #define LED_T1L 650 #define LED_T0H 250 #define LED_T0L 1000 -#define NS_PER_SEC (1000000000L) -#define CYCLES_PER_SEC (F_CPU) -#define NS_PER_CYCLE (NS_PER_SEC / CYCLES_PER_SEC) -#define NS_TO_CYCLES(n) ((n) / NS_PER_CYCLE) - void send_bit_d4(bool bitVal) { if(bitVal) { asm volatile ( @@ -66,14 +60,12 @@ void send_bit_d4(bool bitVal) { } } -void show(void) { - _delay_us((RES / 1000UL) + 1); -} - -void send_value(uint8_t byte) { +void send_value(uint8_t byte, enum Device device) { for(uint8_t b = 0; b < 8; b++) { - send_bit_d4(byte & 0b10000000); - byte <<= 1; + if(device == Device_STATUSLED) { + send_bit_d4(byte & 0b10000000); + byte <<= 1; + } } } @@ -83,7 +75,8 @@ void indicator_leds_set(bool leds[8]) { cli(); for(led_cnt = 0; led_cnt < 8; led_cnt++) - send_value(leds[led_cnt] ? 255 : 0); + send_value(leds[led_cnt] ? 255 : 0, Device_STATUSLED); sei(); show(); } + diff --git a/keyboards/duck/eagle_viper/v2/indicator_leds.h b/keyboards/duck/eagle_viper/v2/indicator_leds.h index c174fa404d..fe66eef6b2 100644 --- a/keyboards/duck/eagle_viper/v2/indicator_leds.h +++ b/keyboards/duck/eagle_viper/v2/indicator_leds.h @@ -1,2 +1 @@ void indicator_leds_set(bool leds[8]); -void show(void); diff --git a/keyboards/duck/eagle_viper/v2/matrix.c b/keyboards/duck/eagle_viper/v2/matrix.c index 7003a7ae00..a6bc563422 100644 --- a/keyboards/duck/eagle_viper/v2/matrix.c +++ b/keyboards/duck/eagle_viper/v2/matrix.c @@ -89,6 +89,9 @@ uint8_t matrix_scan(void) { bool curr_bit = rows & (1<<row); if (prev_bit != curr_bit) { matrix_debouncing[row] ^= ((matrix_row_t)1<<col); + if (debouncing) { + dprint("bounce!: "); dprintf("%02X", debouncing); dprintln(); + } debouncing = DEBOUNCING_DELAY; } } diff --git a/keyboards/duck/eagle_viper/v2/readme.md b/keyboards/duck/eagle_viper/v2/readme.md index 32ad8453ca..31f70b93b9 100644 --- a/keyboards/duck/eagle_viper/v2/readme.md +++ b/keyboards/duck/eagle_viper/v2/readme.md @@ -11,6 +11,8 @@ Make example for this keyboard (after setting up your build environment): make eagle_viper/v2:default +**Reset Key:** To put the Eagle/Viper V2 into reset, hold caps lock key (`K2A`) while plugging in. + See [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) then the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. ## Hardware Notes diff --git a/keyboards/duck/eagle_viper/v2/rules.mk b/keyboards/duck/eagle_viper/v2/rules.mk index 64d839fcbd..1abd7d9413 100644 --- a/keyboards/duck/eagle_viper/v2/rules.mk +++ b/keyboards/duck/eagle_viper/v2/rules.mk @@ -50,23 +50,23 @@ OPT_DEFS += -DBOOTLOADER_SIZE=4096 # Build Options # change yes to no to disable # -BOOTMAGIC_ENABLE ?= no # Virtual DIP switch configuration(+1000) -MOUSEKEY_ENABLE ?= no # Mouse keys(+4700) -EXTRAKEY_ENABLE ?= yes # Audio control and System control(+450) -CONSOLE_ENABLE ?= no # Console for debug(+400) -COMMAND_ENABLE ?= yes # Commands for debug and configuration +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = no # Mouse keys(+4700) +EXTRAKEY_ENABLE = yes # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration # Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE -SLEEP_LED_ENABLE ?= no # Breathing sleep LED during USB suspend +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend # if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work -NKRO_ENABLE ?= yes # USB Nkey Rollover -BACKLIGHT_ENABLE ?= yes # Enable keyboard backlight functionality on B7 by default -MIDI_ENABLE ?= no # MIDI support (+2400 to 4200, depending on config) -UNICODE_ENABLE ?= no # Unicode -BLUETOOTH_ENABLE ?= no # Enable Bluetooth with the Adafruit EZ-Key HID -AUDIO_ENABLE ?= no # Audio output on port C6 -FAUXCLICKY_ENABLE ?= no # Use buzzer to emulate clicky switches +NKRO_ENABLE = yes # USB Nkey Rollover +BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality on B7 by default +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches RGBLIGHT_ENABLE = yes CUSTOM_MATRIX = yes SRC += indicator_leds.c \ - matrix.c + matrix.c duck_led/duck_led.c diff --git a/keyboards/duck/eagle_viper/v2/v2.h b/keyboards/duck/eagle_viper/v2/v2.h index d149471ba3..7fefcf770c 100644 --- a/keyboards/duck/eagle_viper/v2/v2.h +++ b/keyboards/duck/eagle_viper/v2/v2.h @@ -13,8 +13,7 @@ * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef V2_H -#define V2_H +#pragma once #include "quantum.h" @@ -78,4 +77,3 @@ #define LAYOUT_eagle LAYOUT_60_ansi -#endif diff --git a/keyboards/duck/jetfire/config.h b/keyboards/duck/jetfire/config.h index 4bc535b192..774e284918 100644 --- a/keyboards/duck/jetfire/config.h +++ b/keyboards/duck/jetfire/config.h @@ -48,6 +48,10 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. /* Debounce reduces chatter (unintended double-presses) - set 0 if debouncing is not needed */ #define DEBOUNCING_DELAY 5 +/* Set to top left most key */ +#define BOOTMAGIC_LITE_ROW 5 +#define BOOTMAGIC_LITE_COLUMN 10 + /* If defined, GRAVE_ESC will always act as ESC when CTRL is held. * This is userful for the Windows task manager shortcut (ctrl+shift+esc). */ diff --git a/keyboards/duck/jetfire/backlight_led.c b/keyboards/duck/jetfire/indicator_leds.c index 7e9dca6e9f..7dbdb1ff79 100644 --- a/keyboards/duck/jetfire/backlight_led.c +++ b/keyboards/duck/jetfire/indicator_leds.c @@ -17,21 +17,13 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #include <stdbool.h> #include <util/delay.h> #include <stdint.h> -#include "backlight_led.h" +#include "indicator_leds.h" #include "quantum.h" -// #include "led.h" - -#define T1H 900 -#define T1L 600 -#define T0H 400 -#define T0L 900 -#define RES 6000 - -#define NS_PER_SEC (1000000000L) -#define CYCLES_PER_SEC (F_CPU) -#define NS_PER_CYCLE (NS_PER_SEC / CYCLES_PER_SEC) -#define NS_TO_CYCLES(n) ((n) / NS_PER_CYCLE) +#define LED_T1H 900 +#define LED_T1L 600 +#define LED_T0H 400 +#define LED_T0L 900 void send_bit_d4(bool bitVal) { @@ -48,8 +40,8 @@ void send_bit_d4(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T1H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T1L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T1H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T1L) - 2)); } else { asm volatile ( "sbi %[port], %[bit] \n\t" @@ -63,8 +55,8 @@ void send_bit_d4(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T0H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T0L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T0H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T0L) - 2)); } } @@ -83,8 +75,8 @@ void send_bit_d6(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (6), - [onCycles] "I" (NS_TO_CYCLES(T1H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T1L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T1H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T1L) - 2)); } else { asm volatile ( "sbi %[port], %[bit] \n\t" @@ -98,20 +90,15 @@ void send_bit_d6(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (6), - [onCycles] "I" (NS_TO_CYCLES(T0H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T0L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T0H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T0L) - 2)); } } -void show(void) -{ - _delay_us((RES / 1000UL) + 1); -} - void send_value(uint8_t byte, enum Device device) { for(uint8_t b = 0; b < 8; b++) { - if(device == Device_STATELED) { + if(device == Device_STATUSLED) { send_bit_d4(byte & 0b10000000); } if(device == Device_PCBRGB) { @@ -123,7 +110,7 @@ void send_value(uint8_t byte, enum Device device) void send_color(uint8_t r, uint8_t g, uint8_t b, enum Device device) { - send_value(g, device); send_value(r, device); + send_value(g, device); send_value(b, device); } diff --git a/keyboards/duck/jetfire/backlight_led.h b/keyboards/duck/jetfire/indicator_leds.h index 36d8d9aa9b..695e1db6d4 100644 --- a/keyboards/duck/jetfire/backlight_led.h +++ b/keyboards/duck/jetfire/indicator_leds.h @@ -1,10 +1,6 @@ -#ifndef BACKLIGHT_LED_H -#define BACKLIGHT_LED_H +#pragma once -enum Device { - Device_PCBRGB, - Device_STATELED -}; +#include "duck_led/duck_led.h" void backlight_init_ports(void); void backlight_set_state(bool cfg[7]); @@ -13,6 +9,3 @@ void backlight_toggle_rgb(bool enabled); void backlight_set_rgb(uint8_t cfg[17][3]); void backlight_set(uint8_t level); void send_color(uint8_t r, uint8_t g, uint8_t b, enum Device device); -void show(void); - -#endif diff --git a/keyboards/duck/jetfire/jetfire.c b/keyboards/duck/jetfire/jetfire.c index 81bdb95ba1..0662489c6f 100644 --- a/keyboards/duck/jetfire/jetfire.c +++ b/keyboards/duck/jetfire/jetfire.c @@ -14,9 +14,9 @@ * along with this program. If not, see <http://www.gnu.org/licenses/>. */ #include "jetfire.h" -#include "backlight_led.h" +#include "indicator_leds.h" -enum backlight_level { +enum BACKLIGHT_AREAS { BACKLIGHT_ALPHA = 0b0000001, BACKLIGHT_MOD = 0b0000010, BACKLIGHT_FROW = 0b0000100, @@ -137,15 +137,15 @@ void backlight_update_state() send_color(backlight_state_led & (1<<STATE_LED_SCROLL_LOCK) ? 255 : 0, backlight_state_led & (1<<STATE_LED_CAPS_LOCK) ? 255 : 0, backlight_state_led & (1<<STATE_LED_NUM_LOCK) ? 255 : 0, - Device_STATELED); + Device_STATUSLED); send_color(backlight_state_led & (1<<STATE_LED_LAYER_1) ? 255 : 0, backlight_state_led & (1<<STATE_LED_LAYER_2) ? 255 : 0, backlight_state_led & (1<<STATE_LED_LAYER_0) ? 255 : 0, - Device_STATELED); + Device_STATUSLED); send_color(backlight_state_led & (1<<STATE_LED_LAYER_4) ? 255 : 0, backlight_state_led & (1<<STATE_LED_LAYER_3) ? 255 : 0, 0, - Device_STATELED); + Device_STATUSLED); sei(); show(); } diff --git a/keyboards/duck/jetfire/jetfire.h b/keyboards/duck/jetfire/jetfire.h index 9ce1406f0a..3c4834bce4 100644 --- a/keyboards/duck/jetfire/jetfire.h +++ b/keyboards/duck/jetfire/jetfire.h @@ -13,8 +13,7 @@ * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef JETFIRE_H -#define JETFIRE_H +#pragma once #include "quantum.h" @@ -57,4 +56,3 @@ { K0A, K0B, K0C, KC_NO,KC_NO,KC_NO,KC_NO,KC_NO, K0I, KC_NO,KC_NO,KC_NO, K0M, K0N, K0O, K0P, K0Q, K0R, K0S, KC_NO } \ } -#endif diff --git a/keyboards/duck/jetfire/readme.md b/keyboards/duck/jetfire/readme.md index 34b351a726..8de2f5ced6 100644 --- a/keyboards/duck/jetfire/readme.md +++ b/keyboards/duck/jetfire/readme.md @@ -9,7 +9,7 @@ Keyboard Maintainer: [MechMerlin](https://github.com/mechmerlin) Hardware Supported: Duck Jetfire PCB Hardware Availability: [Geekhack GB](https://geekhack.org/index.php?topic=92708.0) -To get into bootloader mode, hold the top top most key above the 2 navigation keys while connecting the USB cable. +**Reset Key:** To put the Jetfire into reset, hold top most key above the 2 navigation keys (`K5P`) while plugging in. Make example for this keyboard (after setting up your build environment): diff --git a/keyboards/duck/jetfire/rules.mk b/keyboards/duck/jetfire/rules.mk index 8e05516df3..c708593293 100644 --- a/keyboards/duck/jetfire/rules.mk +++ b/keyboards/duck/jetfire/rules.mk @@ -51,7 +51,7 @@ OPT_DEFS += -DBOOTLOADER_SIZE=4096 # Build Options # change yes to no to disable # -BOOTMAGIC_ENABLE = no # Virtual DIP switch configuration(+1000) +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) MOUSEKEY_ENABLE = no # Mouse keys(+4700) EXTRAKEY_ENABLE = no # Audio control and System control(+450) CONSOLE_ENABLE = yes # Console for debug(+400) @@ -70,5 +70,5 @@ FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches HD44780_ENABLE = no # Enable support for HD44780 based LCDs (+400) CUSTOM_MATRIX = yes -SRC += backlight_led.c \ - matrix.c +SRC += indicator_leds.c \ + matrix.c duck_led/duck_led.c diff --git a/keyboards/duck/lightsaver/config.h b/keyboards/duck/lightsaver/config.h index 9e3a08fbd1..d302fb3953 100644 --- a/keyboards/duck/lightsaver/config.h +++ b/keyboards/duck/lightsaver/config.h @@ -15,8 +15,7 @@ You should have received a copy of the GNU General Public License along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef CONFIG_H -#define CONFIG_H +#pragma once #include "config_common.h" @@ -44,6 +43,9 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_DI_PIN D6 #define RGBLED_NUM 17 +/* Set to top left most key */ +#define BOOTMAGIC_LITE_ROW 5 +#define BOOTMAGIC_LITE_COLUMN 10 + #define TAPPING_TERM 200 -#endif diff --git a/keyboards/duck/lightsaver/indicator_leds.c b/keyboards/duck/lightsaver/indicator_leds.c index 0a54e151e1..5d2e1ad9f6 100644 --- a/keyboards/duck/lightsaver/indicator_leds.c +++ b/keyboards/duck/lightsaver/indicator_leds.c @@ -18,17 +18,12 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #include <avr/io.h> #include <stdbool.h> #include <util/delay.h> +#include "duck_led/duck_led.h" -#define T1H 900 -#define T1L 600 -#define T0H 400 -#define T0L 900 -#define RES 6000 - -#define NS_PER_SEC (1000000000L) -#define CYCLES_PER_SEC (F_CPU) -#define NS_PER_CYCLE (NS_PER_SEC / CYCLES_PER_SEC) -#define NS_TO_CYCLES(n) ((n) / NS_PER_CYCLE) +#define LED_T1H 900 +#define LED_T1L 600 +#define LED_T0H 400 +#define LED_T0L 900 void send_bit_d4(bool bitVal) { if(bitVal) { @@ -44,8 +39,8 @@ void send_bit_d4(bool bitVal) { :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T1H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T1L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T1H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T1L) - 2)); } else { asm volatile ( "sbi %[port], %[bit] \n\t" @@ -59,33 +54,31 @@ void send_bit_d4(bool bitVal) { :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T0H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T0L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T0H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T0L) - 2)); } } -void show(void) { - _delay_us((RES / 1000UL) + 1); -} - -void send_value(uint8_t byte) { +void send_value(uint8_t byte, enum Device device) { for(uint8_t b = 0; b < 8; b++) { - send_bit_d4(byte & 0b10000000); - byte <<= 1; + if(device == Device_STATUSLED) { + send_bit_d4(byte & 0b10000000); + byte <<= 1; + } } } -void send_color(uint8_t r, uint8_t g, uint8_t b) { - send_value(g); - send_value(r); - send_value(b); +void send_color(uint8_t r, uint8_t g, uint8_t b, enum Device device) { + send_value(r, device); + send_value(g, device); + send_value(b, device); } void indicator_leds_set(bool leds[8]) { cli(); - send_color(leds[1] ? 255 : 0, leds[2] ? 255 : 0, leds[0] ? 255 : 0); - send_color(leds[4] ? 255 : 0, leds[5] ? 255 : 0, leds[3] ? 255 : 0); - send_color(leds[6] ? 255 : 0, leds[7] ? 255 : 0, 0); + send_color(leds[1] ? 255 : 0, leds[2] ? 255 : 0, leds[0] ? 255 : 0, Device_STATUSLED); + send_color(leds[4] ? 255 : 0, leds[5] ? 255 : 0, leds[3] ? 255 : 0, Device_STATUSLED); + send_color(leds[6] ? 255 : 0, leds[7] ? 255 : 0, 0, Device_STATUSLED); sei(); show(); } diff --git a/keyboards/duck/lightsaver/lightsaver.h b/keyboards/duck/lightsaver/lightsaver.h index 19fcf36bac..1e1185713b 100644 --- a/keyboards/duck/lightsaver/lightsaver.h +++ b/keyboards/duck/lightsaver/lightsaver.h @@ -13,8 +13,7 @@ * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef LIGHTSAVER_H -#define LIGHTSAVER_H +#pragma once #include "quantum.h" @@ -37,4 +36,3 @@ /* 5 */ { K0A, K0B, K0C, NO, NO, NO, NO, NO, K0I, NO, K0K, NO, K0M, K0N, K0O, K0P, K0Q, K0R } \ } -#endif diff --git a/keyboards/duck/lightsaver/matrix.c b/keyboards/duck/lightsaver/matrix.c index a07cdd0d16..543205c0b7 100644 --- a/keyboards/duck/lightsaver/matrix.c +++ b/keyboards/duck/lightsaver/matrix.c @@ -87,6 +87,9 @@ uint8_t matrix_scan(void) { bool curr_bit = rows & (1<<row); if (prev_bit != curr_bit) { matrix_debouncing[row] ^= ((matrix_row_t)1<<col); + if (debouncing) { + dprint("bounce!: "); dprintf("%02X", debouncing); dprintln(); + } debouncing = DEBOUNCING_DELAY; } } diff --git a/keyboards/duck/lightsaver/rules.mk b/keyboards/duck/lightsaver/rules.mk index d95dbd9603..bc7b901b49 100644 --- a/keyboards/duck/lightsaver/rules.mk +++ b/keyboards/duck/lightsaver/rules.mk @@ -50,23 +50,23 @@ OPT_DEFS += -DBOOTLOADER_SIZE=4096 # Build Options # change yes to no to disable # -BOOTMAGIC_ENABLE ?= no # Virtual DIP switch configuration(+1000) -MOUSEKEY_ENABLE ?= no # Mouse keys(+4700) -EXTRAKEY_ENABLE ?= no # Audio control and System control(+450) -CONSOLE_ENABLE ?= no # Console for debug(+400) -COMMAND_ENABLE ?= yes # Commands for debug and configuration +BOOTMAGIC_ENABLE = no # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = no # Mouse keys(+4700) +EXTRAKEY_ENABLE = no # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration # Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE -SLEEP_LED_ENABLE ?= no # Breathing sleep LED during USB suspend +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend # if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work -NKRO_ENABLE ?= yes # USB Nkey Rollover -BACKLIGHT_ENABLE ?= yes # Enable keyboard backlight functionality on B7 by default -MIDI_ENABLE ?= no # MIDI support (+2400 to 4200, depending on config) -UNICODE_ENABLE ?= no # Unicode -BLUETOOTH_ENABLE ?= no # Enable Bluetooth with the Adafruit EZ-Key HID -AUDIO_ENABLE ?= no # Audio output on port C6 -FAUXCLICKY_ENABLE ?= no # Use buzzer to emulate clicky switches +NKRO_ENABLE = yes # USB Nkey Rollover +BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality on B7 by default +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches RGBLIGHT_ENABLE = yes CUSTOM_MATRIX = yes SRC += indicator_leds.c \ - matrix.c + matrix.c duck_led/duck_led.c diff --git a/keyboards/duck/octagon/v1/config.h b/keyboards/duck/octagon/v1/config.h index 5400c53ef2..d818cb6223 100644 --- a/keyboards/duck/octagon/v1/config.h +++ b/keyboards/duck/octagon/v1/config.h @@ -15,8 +15,7 @@ You should have received a copy of the GNU General Public License along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef CONFIG_H -#define CONFIG_H +#pragma once #include "config_common.h" @@ -44,6 +43,8 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_DI_PIN D6 #define RGBLED_NUM 17 -#define TAPPING_TERM 200 +/* Set to top left most key */ +#define BOOTMAGIC_LITE_ROW 5 +#define BOOTMAGIC_LITE_COLUMN 10 -#endif +#define TAPPING_TERM 200 diff --git a/keyboards/duck/octagon/v1/matrix.c b/keyboards/duck/octagon/v1/matrix.c index 8555349943..233404ed30 100644 --- a/keyboards/duck/octagon/v1/matrix.c +++ b/keyboards/duck/octagon/v1/matrix.c @@ -84,6 +84,9 @@ uint8_t matrix_scan(void) { bool curr_bit = rows & (1<<row); if (prev_bit != curr_bit) { matrix_debouncing[row] ^= ((matrix_row_t)1<<col); + if (debouncing) { + dprint("bounce!: "); dprintf("%02X", debouncing); dprintln(); + } debouncing = DEBOUNCING_DELAY; } } diff --git a/keyboards/duck/octagon/v1/rules.mk b/keyboards/duck/octagon/v1/rules.mk index a08695b504..66d2c8defc 100644 --- a/keyboards/duck/octagon/v1/rules.mk +++ b/keyboards/duck/octagon/v1/rules.mk @@ -50,21 +50,21 @@ OPT_DEFS += -DBOOTLOADER_SIZE=4096 # Build Options # change yes to no to disable # -BOOTMAGIC_ENABLE ?= no # Virtual DIP switch configuration(+1000) -MOUSEKEY_ENABLE ?= no # Mouse keys(+4700) -EXTRAKEY_ENABLE ?= no # Audio control and System control(+450) -CONSOLE_ENABLE ?= no # Console for debug(+400) -COMMAND_ENABLE ?= yes # Commands for debug and configuration +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = no # Mouse keys(+4700) +EXTRAKEY_ENABLE = no # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration # Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE -SLEEP_LED_ENABLE ?= no # Breathing sleep LED during USB suspend +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend # if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work -NKRO_ENABLE ?= yes # USB Nkey Rollover -BACKLIGHT_ENABLE ?= yes # Enable keyboard backlight functionality on B7 by default -MIDI_ENABLE ?= no # MIDI support (+2400 to 4200, depending on config) -UNICODE_ENABLE ?= no # Unicode -BLUETOOTH_ENABLE ?= no # Enable Bluetooth with the Adafruit EZ-Key HID -AUDIO_ENABLE ?= no # Audio output on port C6 -FAUXCLICKY_ENABLE ?= no # Use buzzer to emulate clicky switches +NKRO_ENABLE = yes # USB Nkey Rollover +BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality on B7 by default +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches RGBLIGHT_ENABLE = yes CUSTOM_MATRIX = yes diff --git a/keyboards/duck/octagon/v1/v1.h b/keyboards/duck/octagon/v1/v1.h index d89188f22c..471a91a332 100644 --- a/keyboards/duck/octagon/v1/v1.h +++ b/keyboards/duck/octagon/v1/v1.h @@ -13,8 +13,7 @@ * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef V1_H -#define V1_H +#pragma once #include "quantum.h" @@ -49,4 +48,3 @@ { K1A, K1C, K1D, K1E, K1F, K1G, K1H, K1I, K1J, K1K, K1L, KC_NO, K1M, K1N, KC_NO, K1P }, \ { K0A, K0B, K0C, KC_NO, KC_NO, K0G, KC_NO, KC_NO, K0J, K0K, K0L, KC_NO, K0M, K0N, KC_NO, K0P } \ } -#endif diff --git a/keyboards/duck/octagon/v2/config.h b/keyboards/duck/octagon/v2/config.h index 78d18d3bb3..4aab587f66 100644 --- a/keyboards/duck/octagon/v2/config.h +++ b/keyboards/duck/octagon/v2/config.h @@ -15,8 +15,7 @@ You should have received a copy of the GNU General Public License along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef CONFIG_H -#define CONFIG_H +#pragma once #include "config_common.h" @@ -44,6 +43,9 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_DI_PIN D6 #define RGBLED_NUM 17 +/* Set to top left most key */ +#define BOOTMAGIC_LITE_ROW 5 +#define BOOTMAGIC_LITE_COLUMN 10 + #define TAPPING_TERM 200 -#endif diff --git a/keyboards/duck/octagon/v2/indicator_leds.c b/keyboards/duck/octagon/v2/indicator_leds.c index c24509f514..116306fb71 100644 --- a/keyboards/duck/octagon/v2/indicator_leds.c +++ b/keyboards/duck/octagon/v2/indicator_leds.c @@ -20,16 +20,10 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #include <util/delay.h> #include "indicator_leds.h" -#define T1H 900 -#define T1L 600 -#define T0H 400 -#define T0L 900 -#define RES 6000 - -#define NS_PER_SEC (1000000000L) -#define CYCLES_PER_SEC (F_CPU) -#define NS_PER_CYCLE (NS_PER_SEC / CYCLES_PER_SEC) -#define NS_TO_CYCLES(n) ((n) / NS_PER_CYCLE) +#define LED_T1H 900 +#define LED_T1L 600 +#define LED_T0H 400 +#define LED_T0L 900 void send_bit_d4(bool bitVal) { if(bitVal) { @@ -45,8 +39,8 @@ void send_bit_d4(bool bitVal) { :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T1H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T1L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T1H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T1L) - 2)); } else { asm volatile ( "sbi %[port], %[bit] \n\t" @@ -60,8 +54,8 @@ void send_bit_d4(bool bitVal) { :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (4), - [onCycles] "I" (NS_TO_CYCLES(T0H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T0L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T0H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T0L) - 2)); } } @@ -80,8 +74,8 @@ void send_bit_d6(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (6), - [onCycles] "I" (NS_TO_CYCLES(T1H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T1L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T1H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T1L) - 2)); } else { asm volatile ( "sbi %[port], %[bit] \n\t" @@ -95,15 +89,11 @@ void send_bit_d6(bool bitVal) :: [port] "I" (_SFR_IO_ADDR(PORTD)), [bit] "I" (6), - [onCycles] "I" (NS_TO_CYCLES(T0H) - 2), - [offCycles] "I" (NS_TO_CYCLES(T0L) - 2)); + [onCycles] "I" (NS_TO_CYCLES(LED_T0H) - 2), + [offCycles] "I" (NS_TO_CYCLES(LED_T0L) - 2)); } } -void show(void) { - _delay_us((RES / 1000UL) + 1); -} - void send_value(uint8_t byte, enum Device device) { for(uint8_t b = 0; b < 8; b++) { if(device == Device_STATUSLED) { @@ -117,8 +107,8 @@ void send_value(uint8_t byte, enum Device device) { } void send_color(uint8_t r, uint8_t g, uint8_t b, enum Device device) { - send_value(g, device); send_value(r, device); + send_value(g, device); send_value(b, device); } diff --git a/keyboards/duck/octagon/v2/indicator_leds.h b/keyboards/duck/octagon/v2/indicator_leds.h index 9bb2c8ced9..ad3ec54f52 100644 --- a/keyboards/duck/octagon/v2/indicator_leds.h +++ b/keyboards/duck/octagon/v2/indicator_leds.h @@ -1,11 +1,7 @@ -enum Device { - Device_PCBRGB, - Device_STATUSLED -}; +#include "duck_led/duck_led.h" void indicator_leds_set(bool leds[8]); void backlight_toggle_rgb(bool enabled); void backlight_set_rgb(uint8_t cfg[17][3]); void backlight_init_ports(void); void send_color(uint8_t r, uint8_t g, uint8_t b, enum Device device); -void show(void);
\ No newline at end of file diff --git a/keyboards/duck/octagon/v2/matrix.c b/keyboards/duck/octagon/v2/matrix.c index a63a37640f..e6e7046b45 100644 --- a/keyboards/duck/octagon/v2/matrix.c +++ b/keyboards/duck/octagon/v2/matrix.c @@ -89,6 +89,9 @@ uint8_t matrix_scan(void) { bool curr_bit = rows & (1<<row); if (prev_bit != curr_bit) { matrix_debouncing[row] ^= ((matrix_row_t)1<<col); + if (debouncing) { + dprint("bounce!: "); dprintf("%02X", debouncing); dprintln(); + } debouncing = DEBOUNCING_DELAY; } } diff --git a/keyboards/duck/octagon/v2/readme.md b/keyboards/duck/octagon/v2/readme.md index b409454fc6..cc9474fc93 100644 --- a/keyboards/duck/octagon/v2/readme.md +++ b/keyboards/duck/octagon/v2/readme.md @@ -11,6 +11,8 @@ Make example for this keyboard (after setting up your build environment): make octagon/v2:default +**Reset Key:** To put the Octagon V2 into reset, hold the top right most key (`K5Q`) while plugging in. + See [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) then the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. ## Hardware Notes diff --git a/keyboards/duck/octagon/v2/rules.mk b/keyboards/duck/octagon/v2/rules.mk index 37f3938d93..c403247074 100644 --- a/keyboards/duck/octagon/v2/rules.mk +++ b/keyboards/duck/octagon/v2/rules.mk @@ -50,25 +50,25 @@ OPT_DEFS += -DBOOTLOADER_SIZE=4096 # Build Options # change yes to no to disable # -BOOTMAGIC_ENABLE ?= no # Virtual DIP switch configuration(+1000) -MOUSEKEY_ENABLE ?= no # Mouse keys(+4700) -EXTRAKEY_ENABLE ?= no # Audio control and System control(+450) -CONSOLE_ENABLE ?= no # Console for debug(+400) -COMMAND_ENABLE ?= yes # Commands for debug and configuration +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = no # Mouse keys(+4700) +EXTRAKEY_ENABLE = no # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration # Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE -SLEEP_LED_ENABLE ?= no # Breathing sleep LED during USB suspend +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend # if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work -NKRO_ENABLE ?= yes # USB Nkey Rollover -BACKLIGHT_ENABLE ?= yes # Enable keyboard backlight functionality on B7 by default -MIDI_ENABLE ?= no # MIDI support (+2400 to 4200, depending on config) -UNICODE_ENABLE ?= no # Unicode -BLUETOOTH_ENABLE ?= no # Enable Bluetooth with the Adafruit EZ-Key HID -AUDIO_ENABLE ?= no # Audio output on port C6 -FAUXCLICKY_ENABLE ?= no # Use buzzer to emulate clicky switches +NKRO_ENABLE = yes # USB Nkey Rollover +BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality on B7 by default +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches RGBLIGHT_ENABLE = yes CUSTOM_MATRIX = yes SRC += indicator_leds.c \ - matrix.c + matrix.c duck_led/duck_led.c LAYOUTS = 75_ansi diff --git a/keyboards/duck/octagon/v2/v2.h b/keyboards/duck/octagon/v2/v2.h index 1487b3640a..d60631009c 100644 --- a/keyboards/duck/octagon/v2/v2.h +++ b/keyboards/duck/octagon/v2/v2.h @@ -13,8 +13,7 @@ * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. */ -#ifndef V2_H -#define V2_H +#pragma once #include "quantum.h" @@ -49,4 +48,3 @@ { K1A, KC_NO, K1C, K1D, K1E, K1F, K1G, K1H, K1I, K1J, K1K, KC_NO, K1M, K1N, K1O, K1P, KC_NO }, \ { K0A, K0B, K0C, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, K0J, KC_NO, K0K, K0L, K0M, K0N, K0O, K0P, KC_NO } \ } -#endif diff --git a/keyboards/dztech/dz40rgb/config.h b/keyboards/dztech/dz40rgb/config.h index 5b9f6dc089..0d6c4ae39a 100644 --- a/keyboards/dztech/dz40rgb/config.h +++ b/keyboards/dztech/dz40rgb/config.h @@ -22,7 +22,6 @@ #define DEBOUNCE 3 #define RGB_DISABLE_AFTER_TIMEOUT 0 // number of ticks to wait until disabling effects #define RGB_DISABLE_WHEN_USB_SUSPENDED false // turn off effects when suspended -#define RGB_MATRIX_SKIP_FRAMES 10 #define RGB_MATRIX_KEYPRESSES #define DISABLE_RGB_MATRIX_SPLASH #define DISABLE_RGB_MATRIX_MULTISPLASH diff --git a/keyboards/dztech/dz60rgb/config.h b/keyboards/dztech/dz60rgb/config.h index 19f32440ea..f43b39810f 100644 --- a/keyboards/dztech/dz60rgb/config.h +++ b/keyboards/dztech/dz60rgb/config.h @@ -23,8 +23,9 @@ #define DEBOUNCE 3 #define RGB_DISABLE_AFTER_TIMEOUT 0 // number of ticks to wait until disabling effects #define RGB_DISABLE_WHEN_USB_SUSPENDED false // turn off effects when suspended -#define RGB_MATRIX_SKIP_FRAMES 10 #define RGB_MATRIX_KEYPRESSES +#define RGB_MATRIX_LED_PROCESS_LIMIT 4 +#define RGB_MATRIX_LED_FLUSH_LIMIT 26 #define DISABLE_RGB_MATRIX_SPLASH #define DISABLE_RGB_MATRIX_MULTISPLASH #define DISABLE_RGB_MATRIX_SOLID_MULTISPLASH diff --git a/keyboards/ergodox_ez/config.h b/keyboards/ergodox_ez/config.h index 096368f7ab..a75edd4154 100644 --- a/keyboards/ergodox_ez/config.h +++ b/keyboards/ergodox_ez/config.h @@ -109,7 +109,6 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define DRIVER_1_LED_TOTAL 24 #define DRIVER_2_LED_TOTAL 24 #define DRIVER_LED_TOTAL DRIVER_1_LED_TOTAL + DRIVER_2_LED_TOTAL -#define RGB_MATRIX_SKIP_FRAMES 10 // #define RGBLIGHT_COLOR_LAYER_0 0x00, 0x00, 0xFF /* #define RGBLIGHT_COLOR_LAYER_1 0x00, 0x00, 0xFF */ diff --git a/keyboards/handwired/tennie/config.h b/keyboards/handwired/tennie/config.h new file mode 100644 index 0000000000..b50886cdc8 --- /dev/null +++ b/keyboards/handwired/tennie/config.h @@ -0,0 +1,240 @@ +/* +Copyright 2018 Jack Hildebrandt + +This program is free software: you can redistribute it and/or modify +it under the terms of the GNU General Public License as published by +the Free Software Foundation, either version 2 of the License, or +(at your option) any later version. + +This program is distributed in the hope that it will be useful, +but WITHOUT ANY WARRANTY; without even the implied warranty of +MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the +GNU General Public License for more details. + +You should have received a copy of the GNU General Public License +along with this program. If not, see <http://www.gnu.org/licenses/>. +*/ + +#pragma once + +#include "config_common.h" + +/* USB Device descriptor parameter */ +#define VENDOR_ID 0x1EEE +#define PRODUCT_ID 0x1313 +#define DEVICE_VER 0x0001 +#define MANUFACTURER Jsck +#define PRODUCT Tennie +#define DESCRIPTION Ten key macropad + +/* key matrix size */ +#define MATRIX_ROWS 3 +#define MATRIX_COLS 4 + +/* + * Keyboard Matrix Assignments + * + * Change this to how you wired your keyboard + * COLS: AVR pins used for columns, left to right + * ROWS: AVR pins used for rows, top to bottom + * DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode) + * ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode) + * +*/ +#define MATRIX_ROW_PINS { C6, D4, D0} +#define MATRIX_COL_PINS { D7, E6, B4, B5 } +#define UNUSED_PINS { B1, B2, B3, B6, F4, F5, F6, F7 D1} + +/* COL2ROW, ROW2COL, or CUSTOM_MATRIX */ +#define DIODE_DIRECTION COL2ROW + +/* + * Split Keyboard specific options, make sure you have 'SPLIT_KEYBOARD = yes' in your rules.mk, and define SOFT_SERIAL_PIN. + */ +//#define SOFT_SERIAL_PIN D0 // or D1, D2, D3, E6 + +// #define BACKLIGHT_PIN B7 +// #define BACKLIGHT_BREATHING +// #define BACKLIGHT_LEVELS 3 +#define RGBW +#define RGB_DI_PIN D1 +#ifdef RGB_DI_PIN + #define RGBLED_NUM 1 + #define RGBLIGHT_HUE_STEP 8 + #define RGBLIGHT_SAT_STEP 8 + #define RGBLIGHT_VAL_STEP 8 + #define RGBLIGHT_LIMIT_VAL 255 /* The maximum brightness level */ +// #define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */ +// /*== all animations enable ==*/ + #define RGBLIGHT_ANIMATIONS +// /*== or choose animations ==*/ +// #define RGBLIGHT_EFFECT_BREATHING +// #define RGBLIGHT_EFFECT_RAINBOW_MOOD +// #define RGBLIGHT_EFFECT_RAINBOW_SWIRL +// #define RGBLIGHT_EFFECT_SNAKE +// #define RGBLIGHT_EFFECT_KNIGHT +// #define RGBLIGHT_EFFECT_CHRISTMAS +// #define RGBLIGHT_EFFECT_STATIC_GRADIENT +// #define RGBLIGHT_EFFECT_RGB_TEST +// #define RGBLIGHT_EFFECT_ALTERNATING +#endif + +/* Debounce reduces chatter (unintended double-presses) - set 0 if debouncing is not needed */ +#define DEBOUNCING_DELAY 5 + +/* define if matrix has ghost (lacks anti-ghosting diodes) */ +//#define MATRIX_HAS_GHOST + +/* number of backlight levels */ + +/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */ +#define LOCKING_SUPPORT_ENABLE +/* Locking resynchronize hack */ +#define LOCKING_RESYNC_ENABLE + +/* If defined, GRAVE_ESC will always act as ESC when CTRL is held. + * This is userful for the Windows task manager shortcut (ctrl+shift+esc). + */ +// #define GRAVE_ESC_CTRL_OVERRIDE + +/* + * Force NKRO + * + * Force NKRO (nKey Rollover) to be enabled by default, regardless of the saved + * state in the bootmagic EEPROM settings. (Note that NKRO must be enabled in the + * makefile for this to work.) + * + * If forced on, NKRO can be disabled via magic key (default = LShift+RShift+N) + * until the next keyboard reset. + * + * NKRO may prevent your keystrokes from being detected in the BIOS, but it is + * fully operational during normal computer usage. + * + * For a less heavy-handed approach, enable NKRO via magic key (LShift+RShift+N) + * or via bootmagic (hold SPACE+N while plugging in the keyboard). Once set by + * bootmagic, NKRO mode will always be enabled until it is toggled again during a + * power-up. + * + */ +//#define FORCE_NKRO + +/* + * Magic Key Options + * + * Magic keys are hotkey commands that allow control over firmware functions of + * the keyboard. They are best used in combination with the HID Listen program, + * found here: https://www.pjrc.com/teensy/hid_listen.html + * + * The options below allow the magic key functionality to be changed. This is + * useful if your keyboard/keypad is missing keys and you want magic key support. + * + */ + +/* control how magic key switches layers */ +//#define MAGIC_KEY_SWITCH_LAYER_WITH_FKEYS true +//#define MAGIC_KEY_SWITCH_LAYER_WITH_NKEYS true +//#define MAGIC_KEY_SWITCH_LAYER_WITH_CUSTOM false + +/* override magic key keymap */ +//#define MAGIC_KEY_SWITCH_LAYER_WITH_FKEYS +//#define MAGIC_KEY_SWITCH_LAYER_WITH_NKEYS +//#define MAGIC_KEY_SWITCH_LAYER_WITH_CUSTOM +//#define MAGIC_KEY_HELP1 H +//#define MAGIC_KEY_HELP2 SLASH +//#define MAGIC_KEY_DEBUG D +//#define MAGIC_KEY_DEBUG_MATRIX X +//#define MAGIC_KEY_DEBUG_KBD K +//#define MAGIC_KEY_DEBUG_MOUSE M +//#define MAGIC_KEY_VERSION V +//#define MAGIC_KEY_STATUS S +//#define MAGIC_KEY_CONSOLE C +//#define MAGIC_KEY_LAYER0_ALT1 ESC +//#define MAGIC_KEY_LAYER0_ALT2 GRAVE +//#define MAGIC_KEY_LAYER0 0 +//#define MAGIC_KEY_LAYER1 1 +//#define MAGIC_KEY_LAYER2 2 +//#define MAGIC_KEY_LAYER3 3 +//#define MAGIC_KEY_LAYER4 4 +//#define MAGIC_KEY_LAYER5 5 +//#define MAGIC_KEY_LAYER6 6 +//#define MAGIC_KEY_LAYER7 7 +//#define MAGIC_KEY_LAYER8 8 +//#define MAGIC_KEY_LAYER9 9 +//#define MAGIC_KEY_BOOTLOADER PAUSE +//#define MAGIC_KEY_LOCK CAPS +//#define MAGIC_KEY_EEPROM E +//#define MAGIC_KEY_NKRO N +//#define MAGIC_KEY_SLEEP_LED Z + +/* + * Feature disable options + * These options are also useful to firmware size reduction. + */ + +/* disable debug print */ +//#define NO_DEBUG + +/* disable print */ +//#define NO_PRINT + +/* disable action features */ +//#define NO_ACTION_LAYER +//#define NO_ACTION_TAPPING +//#define NO_ACTION_ONESHOT +//#define NO_ACTION_MACRO +//#define NO_ACTION_FUNCTION + +/* + * MIDI options + */ + +/* Prevent use of disabled MIDI features in the keymap */ +//#define MIDI_ENABLE_STRICT 1 + +/* enable basic MIDI features: + - MIDI notes can be sent when in Music mode is on +*/ +//#define MIDI_BASIC + +/* enable advanced MIDI features: + - MIDI notes can be added to the keymap + - Octave shift and transpose + - Virtual sustain, portamento, and modulation wheel + - etc. +*/ +//#define MIDI_ADVANCED + +/* override number of MIDI tone keycodes (each octave adds 12 keycodes and allocates 12 bytes) */ +//#define MIDI_TONE_KEYCODE_OCTAVES 1 + +/* + * HD44780 LCD Display Configuration + */ +/* +#define LCD_LINES 2 //< number of visible lines of the display +#define LCD_DISP_LENGTH 16 //< visibles characters per line of the display + +#define LCD_IO_MODE 1 //< 0: memory mapped mode, 1: IO port mode + +#if LCD_IO_MODE +#define LCD_PORT PORTB //< port for the LCD lines +#define LCD_DATA0_PORT LCD_PORT //< port for 4bit data bit 0 +#define LCD_DATA1_PORT LCD_PORT //< port for 4bit data bit 1 +#define LCD_DATA2_PORT LCD_PORT //< port for 4bit data bit 2 +#define LCD_DATA3_PORT LCD_PORT //< port for 4bit data bit 3 +#define LCD_DATA0_PIN 4 //< pin for 4bit data bit 0 +#define LCD_DATA1_PIN 5 //< pin for 4bit data bit 1 +#define LCD_DATA2_PIN 6 //< pin for 4bit data bit 2 +#define LCD_DATA3_PIN 7 //< pin for 4bit data bit 3 +#define LCD_RS_PORT LCD_PORT //< port for RS line +#define LCD_RS_PIN 3 //< pin for RS line +#define LCD_RW_PORT LCD_PORT //< port for RW line +#define LCD_RW_PIN 2 //< pin for RW line +#define LCD_E_PORT LCD_PORT //< port for Enable line +#define LCD_E_PIN 1 //< pin for Enable line +#endif +*/ + +/* Bootmagic Lite key configuration */ +//#define BOOTMAGIC_LITE_ROW 0 +//#define BOOTMAGIC_LITE_COLUMN 0 diff --git a/keyboards/handwired/tennie/info.json b/keyboards/handwired/tennie/info.json new file mode 100644 index 0000000000..c036997929 --- /dev/null +++ b/keyboards/handwired/tennie/info.json @@ -0,0 +1,12 @@ +{ + "keyboard_name": "Tennie", + "url": "https://github.com/StoutIEEE/macropad-workshop", + "maintainer": "UW Stout IEEE, Jack Hildebrandt (onemorebyte)", + "width": 4, + "height": 3, + "layouts": { + "LAYOUT": { + "layout": [{"x":0.5, "y":0}, {"x":1.5, "y":0}, {"x":2.5, "y":0}, {"x":0, "y":1}, {"x":1, "y":1}, {"x":2, "y":1}, {"x":3, "y":1}, {"x":0.5, "y":2}, {"x":1.5, "y":2}, {"x":2.5, "y":2}] + } + } +} diff --git a/keyboards/handwired/tennie/keymaps/default/config.h b/keyboards/handwired/tennie/keymaps/default/config.h new file mode 100644 index 0000000000..4496c59100 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/default/config.h @@ -0,0 +1,19 @@ +/* Copyright 2018 REPLACE_WITH_YOUR_NAME + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ + +#pragma once + +// place overrides here diff --git a/keyboards/handwired/tennie/keymaps/default/keymap.c b/keyboards/handwired/tennie/keymaps/default/keymap.c new file mode 100644 index 0000000000..3736841cbb --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/default/keymap.c @@ -0,0 +1,95 @@ +/* Copyright 2018 Jack H. + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include QMK_KEYBOARD_H + +#define TAPPING_TOGGLE 2 + + +// Layer names +#define base 0 +#define shrek 1 +#define ogre 2 +#define tcp 3 + +// Layer Switches +#define KC_SHRK TT(shrek) +#define KC_OGRE TT(ogre) +#define KC_TCP TT(tcp) + + + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + [base] = LAYOUT( + // ┌────────┬────────┬────────┐ + + KC_DEL, KC_SPC, KC_ENT, + + // ├────────┼────────┼────────┼────────┼ + + KC_LEFT, KC_DOWN, KC_UP , KC_RGHT, + + // ├────────┼────────┼────────┼────────┼ + + KC_SHRK, KC_OGRE, KC_TCP + + // └────────┴────────┴────────┘ + ), + [shrek] = LAYOUT( + // ┌────────┬────────┬────────┐ + + KC_MPRV, KC_MPLY, KC_MNXT, + + // ├────────┼────────┼────────┼────────┼ + + KC_BRID, KC_VOLD, KC_VOLU, KC_BRIU, + + // ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + + // └────────┴────────┴────────┘ + ), + [ogre] = LAYOUT( + // ┌────────┬────────┬────────┐ + + RGB_MOD, RGB_TOG, RGB_RMOD, + + // ├────────┼────────┼────────┼────────┼ + + KC_F13, KC_F14, KC_F15, KC_F16, + + // ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + + // └────────┴────────┴────────┘ + ), + [tcp] = LAYOUT( + // ┌────────┬────────┬────────┐ + + KC_WBAK, KC_WREF, KC_WFWD, + + // ├────────┼────────┼────────┼────────┼ + + XXXXXXX, KC_PGDN, KC_PGUP, XXXXXXX, + + // ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + + // └────────┴────────┴────────┘ + ), +}; diff --git a/keyboards/handwired/tennie/keymaps/default/readme.md b/keyboards/handwired/tennie/keymaps/default/readme.md new file mode 100644 index 0000000000..1fbaa7f463 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/default/readme.md @@ -0,0 +1,68 @@ +# Default keymap + +This keymap is to serve as an example of how you could make a multi-layer keymap. + +#### keymap + +``` +[base] = LAYOUT_kc( +// ┌────────┬────────┬────────┐ + + DEL , SPC , ENT , + +// ├────────┼────────┼────────┼────────┼ + + LEFT , DOWN , UP , RGHT , + +// ├────────┼────────┼────────┼────────┼ + + SHRK , OGRE , TCP + +// └────────┴────────┴────────┘ +), +[shrek] = LAYOUT_kc( +// ┌────────┬────────┬────────┐ + + MPRV , MPLY , MNXT , + +// ├────────┼────────┼────────┼────────┼ + + BRID , VOLD , VOLU , BRIU , + +// ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + +// └────────┴────────┴────────┘ +), +[ogre] = LAYOUT_kc( +// ┌────────┬────────┬────────┐ + + RGB_MOD, RGB_TOG, RGB_RMOD + +// ├────────┼────────┼────────┼────────┼ + + F13 , F14 , F15 , F16 , + +// ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + +// └────────┴────────┴────────┘ +), +[tcp] = LAYOUT_kc( +// ┌────────┬────────┬────────┐ + + WBAK , WREF , WFWD , + +// ├────────┼────────┼────────┼────────┼ + + XXXXXXX , PGDN , PGUP , XXXXXXX , + +// ├────────┼────────┼────────┼────────┼ + + _______, _______, _______ + +// └────────┴────────┴────────┘ +), +``` diff --git a/keyboards/handwired/tennie/keymaps/simple-numbpad/config.h b/keyboards/handwired/tennie/keymaps/simple-numbpad/config.h new file mode 100644 index 0000000000..a3c309bb29 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/simple-numbpad/config.h @@ -0,0 +1,19 @@ +/* Copyright 2018 Jack Hildebrandt + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ + +#pragma once + +// place overrides here diff --git a/keyboards/handwired/tennie/keymaps/simple-numbpad/keymap.c b/keyboards/handwired/tennie/keymaps/simple-numbpad/keymap.c new file mode 100644 index 0000000000..7cf29b1188 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/simple-numbpad/keymap.c @@ -0,0 +1,37 @@ +/* Copyright 2018 Jack Hildebrandt + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include QMK_KEYBOARD_H + +// Layer names +#define base 0 + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + [base] = LAYOUT( + // ┌────────┬────────┬────────┐ + + KC_1 , KC_2 , KC_3 , + + // ├────────┼────────┼────────┼────────┼ + + KC_4 , KC_5 , KC_6 , KC_7 , + + // ├────────┼────────┼────────┼────────┼ + + KC_8 , KC_9 , KC_0 + + // └────────┴────────┴────────┘ + ), +}; diff --git a/keyboards/handwired/tennie/keymaps/simple-numbpad/readme.md b/keyboards/handwired/tennie/keymaps/simple-numbpad/readme.md new file mode 100644 index 0000000000..0b3f307501 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/simple-numbpad/readme.md @@ -0,0 +1,18 @@ +# Simple numberpad keymap + +This keymap is to test the soldering work of workshop participants. It can also be used as a simple base for a macropad keymap. +``` +// ┌────────┬────────┬────────┐ + + 1 , 2 , 3 , + +// ├────────┼────────┼────────┼────────┼ + + 4 , 5 , 6 , 7 , + +// ├────────┼────────┼────────┼────────┼ + + 8 , 9 , 0 + +// └────────┴────────┴────────┘ +``` diff --git a/keyboards/handwired/tennie/keymaps/soundboard/config.h b/keyboards/handwired/tennie/keymaps/soundboard/config.h new file mode 100644 index 0000000000..5a1cba7b40 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/soundboard/config.h @@ -0,0 +1,19 @@ +/* Copyright 2018 Jacob Hillebrand + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ + +#pragma once + +// place overrides here diff --git a/keyboards/handwired/tennie/keymaps/soundboard/keymap.c b/keyboards/handwired/tennie/keymaps/soundboard/keymap.c new file mode 100644 index 0000000000..3bceb1feac --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/soundboard/keymap.c @@ -0,0 +1,37 @@ +/* Copyright 2018 Jacob Hillebrand + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include QMK_KEYBOARD_H + +// Layer names +#define base 0 + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + [base] = LAYOUT( + // ┌────────┬────────┬────────┐ + + KC_F13 , KC_F14 , KC_F15 , + + // ├────────┼────────┼────────┼────────┼ + + KC_F16 , KC_F17 , KC_F18 , KC_F19 , + + // ├────────┼────────┼────────┼────────┼ + + KC_F20, KC_F21 , KC_F22 + + // └────────┴────────┴────────┘ + ), +}; diff --git a/keyboards/handwired/tennie/keymaps/soundboard/readme.md b/keyboards/handwired/tennie/keymaps/soundboard/readme.md new file mode 100644 index 0000000000..d05b7117c1 --- /dev/null +++ b/keyboards/handwired/tennie/keymaps/soundboard/readme.md @@ -0,0 +1,19 @@ +# Soundboard keymap + +This keymap binds all keys to function keys 13-22 for something like a +soundboard (ie:[Resanance](https://www.reddit.com/r/discordapp/comments/44ldc1/soundboard_for_discord/)) +``` +// ┌────────┬────────┬────────┐ + + F13 , F14 , F15 , + +// ├────────┼────────┼────────┼────────┼ + + F16 , F17 , F18 , F19 , + +// ├────────┼────────┼────────┼────────┼ + + F20 , F21 , F22 + +// └────────┴────────┴────────┘ +``` diff --git a/keyboards/handwired/tennie/readme.md b/keyboards/handwired/tennie/readme.md new file mode 100644 index 0000000000..bbdbc2b601 --- /dev/null +++ b/keyboards/handwired/tennie/readme.md @@ -0,0 +1,15 @@ +# Tennie + +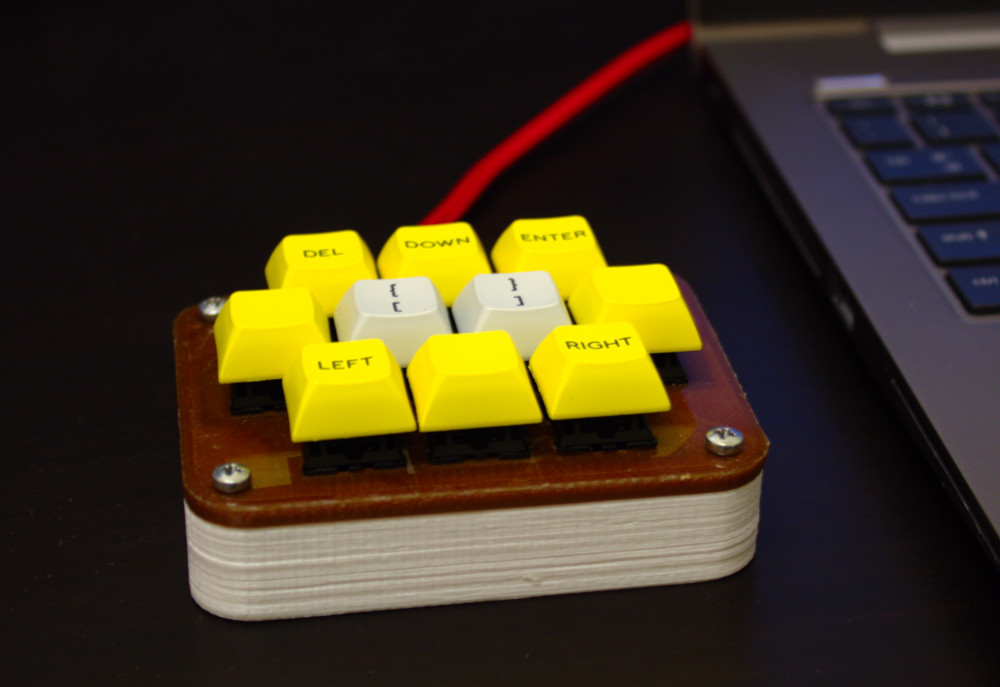 + +A ten key macropad for a club workshop! More information, and build instructions can be found [here](https://github.com/StoutIEEE/macropad-workshop) + +Keyboard Maintainer: [Jack Hildebrandt](https://github.com/onemorebyte), [UW Stout IEEE](https://github.com/StoutIEEE) + +Hardware Supported: ProMicro + +Make example for this keyboard (after setting up your build environment): + + make handwired/tennie:default + +See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs). diff --git a/keyboards/handwired/tennie/rules.mk b/keyboards/handwired/tennie/rules.mk new file mode 100644 index 0000000000..0119dc6fb2 --- /dev/null +++ b/keyboards/handwired/tennie/rules.mk @@ -0,0 +1,80 @@ +# MCU name +MCU = atmega32u4 + +# Processor frequency. +# This will define a symbol, F_CPU, in all source code files equal to the +# processor frequency in Hz. You can then use this symbol in your source code to +# calculate timings. Do NOT tack on a 'UL' at the end, this will be done +# automatically to create a 32-bit value in your source code. +# +# This will be an integer division of F_USB below, as it is sourced by +# F_USB after it has run through any CPU prescalers. Note that this value +# does not *change* the processor frequency - it should merely be updated to +# reflect the processor speed set externally so that the code can use accurate +# software delays. +F_CPU = 16000000 + + +# +# LUFA specific +# +# Target architecture (see library "Board Types" documentation). +ARCH = AVR8 + +# Input clock frequency. +# This will define a symbol, F_USB, in all source code files equal to the +# input clock frequency (before any prescaling is performed) in Hz. This value may +# differ from F_CPU if prescaling is used on the latter, and is required as the +# raw input clock is fed directly to the PLL sections of the AVR for high speed +# clock generation for the USB and other AVR subsections. Do NOT tack on a 'UL' +# at the end, this will be done automatically to create a 32-bit value in your +# source code. +# +# If no clock division is performed on the input clock inside the AVR (via the +# CPU clock adjust registers or the clock division fuses), this will be equal to F_CPU. +F_USB = $(F_CPU) + +# Interrupt driven control endpoint task(+60) +OPT_DEFS += -DINTERRUPT_CONTROL_ENDPOINT + + +# Bootloader selection +# Teensy halfkay +# Pro Micro caterina +# Atmel DFU atmel-dfu +# LUFA DFU lufa-dfu +# QMK DFU qmk-dfu +# atmega32a bootloadHID +BOOTLOADER = caterina + + +# If you don't know the bootloader type, then you can specify the +# Boot Section Size in *bytes* by uncommenting out the OPT_DEFS line +# Teensy halfKay 512 +# Teensy++ halfKay 1024 +# Atmel DFU loader 4096 +# LUFA bootloader 4096 +# USBaspLoader 2048 +# OPT_DEFS += -DBOOTLOADER_SIZE=4096 + + +# Build Options +# change yes to no to disable +# +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = yes # Mouse keys(+4700) +EXTRAKEY_ENABLE = yes # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration +# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend +# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work +NKRO_ENABLE = no # USB Nkey Rollover +BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality on B7 by default +RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches +HD44780_ENABLE = no # Enable support for HD44780 based LCDs (+400) diff --git a/keyboards/handwired/tennie/tennie.c b/keyboards/handwired/tennie/tennie.c new file mode 100644 index 0000000000..e1ceaf153d --- /dev/null +++ b/keyboards/handwired/tennie/tennie.c @@ -0,0 +1,45 @@ +/* Copyright 2018 Jack Hildebrandt + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include "tennie.h" + +void matrix_init_kb(void) { + matrix_init_user(); +} + +void matrix_post_init(void) { + rgblight_enable_noeeprom(); + keyboard_post_init_user(); +} + +void matrix_scan_kb(void) { + // put your looping keyboard code here + // runs every cycle (a lot) + + matrix_scan_user(); +} + +bool process_record_kb(uint16_t keycode, keyrecord_t *record) { + // put your per-action keyboard code here + // runs for every action, just before processing by the firmware + + return process_record_user(keycode, record); +} + +//void led_set_kb(uint8_t usb_led) { + // put your keyboard LED indicator (ex: Caps Lock LED) toggling code here + +// led_set_user(usb_led); +//} diff --git a/keyboards/handwired/tennie/tennie.h b/keyboards/handwired/tennie/tennie.h new file mode 100644 index 0000000000..8ab0d04c52 --- /dev/null +++ b/keyboards/handwired/tennie/tennie.h @@ -0,0 +1,37 @@ +/* Copyright 2018 Jack Hildebrandt + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#pragma once + +#include "quantum.h" + +/* This a shortcut to help you visually see your layout. + * + * The first section contains all of the arguments representing the physical + * layout of the board and position of the keys. + * + * The second converts the arguments into a two-dimensional array which + * represents the switch matrix. + */ +#define LAYOUT( \ + K00, K01, K02, \ + K10, K11, K12, K13, \ + K20, K21, K22 \ +) \ +{ \ + { K00, K01, K02, KC_NO }, \ + { K10, K11, K12, K13 }, \ + { K20, K21, K22, KC_NO }, \ +} diff --git a/keyboards/hs60/v1/config.h b/keyboards/hs60/v1/config.h index 7581e54b61..528f08bb99 100644 --- a/keyboards/hs60/v1/config.h +++ b/keyboards/hs60/v1/config.h @@ -120,7 +120,6 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_DISABLE_AFTER_TIMEOUT 0 // number of ticks to wait until disabling effects #define RGB_DISABLE_WHEN_USB_SUSPENDED false // turn off effects when suspended -#define RGB_MATRIX_SKIP_FRAMES 0 #define RGB_MATRIX_MAXIMUM_BRIGHTNESS 215 #define DRIVER_ADDR_1 0b1110100 diff --git a/keyboards/hs60/v2/config.h b/keyboards/hs60/v2/config.h index b0c56adf89..bbf17251b4 100644 --- a/keyboards/hs60/v2/config.h +++ b/keyboards/hs60/v2/config.h @@ -119,6 +119,11 @@ along with this program. If not, see <http://www.gnu.org/licenses/>. #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_3 0b0010000000000001 #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_4 0b0011110000000111 +#define RGB_BACKLIGHT_CAPS_LOCK_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_1_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_2_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_3_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } + // TODO: refactor with new user EEPROM code (coming soon) #define EEPROM_MAGIC 0x451F #define EEPROM_MAGIC_ADDR 32 diff --git a/keyboards/jj50/keymaps/abstractkb/keymap.c b/keyboards/jj50/keymaps/abstractkb/keymap.c new file mode 100644 index 0000000000..456adeee4b --- /dev/null +++ b/keyboards/jj50/keymaps/abstractkb/keymap.c @@ -0,0 +1,135 @@ +/* +Base Copyright 2017 Luiz Ribeiro <luizribeiro@gmail.com> +Modified 2017 Andrew Novak <ndrw.nvk@gmail.com> +Modified 2018 Wayne Jones (WarmCatUK) <waynekjones@gmail.com> +Modified 2019 AbstractKB + +This program is free software: you can redistribute it and/or modify +it under the terms of the GNU General Public License as published by +the Free Software Foundation, either version 2 of the License, or +(at your option) any later version. + +This program is distributed in the hope that it will be useful, +but WITHOUT ANY WARRANTY; without even the implied warranty of +MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the +GNU General Public License for more details. + +You should have received a copy of the GNU General Public LicensezZZ +along with this program. If not, see <http://www.gnu.org/licenses/>. +*/ + +#include QMK_KEYBOARD_H + +enum layers { + _DEFLT, + _RAISE, + _LOWER, + _FN +}; + +enum custom_keycodes { + MYRGB_TG = SAFE_RANGE +}; + +bool rgbinit = true; +bool rgbon = true; + +const uint8_t RGBLED_RAINBOW_SWIRL_INTERVALS[] PROGMEM = {1,5,5}; //only using the first one + +void keyboard_post_init_user(void) { + rgblight_enable_noeeprom(); + led_set_user(host_keyboard_leds()); +} + +uint32_t layer_state_set_user(uint32_t state) { + switch (biton32(state)) { + case _RAISE: + rgblight_sethsv_noeeprom(240,255,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_STATIC_LIGHT); + break; + case _LOWER: + rgblight_sethsv_noeeprom(0,255,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_STATIC_LIGHT); + break; + case _FN: + rgblight_sethsv_noeeprom(0,255,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_RAINBOW_SWIRL); + break; + default: //_DEFLT + rgblight_sethsv_noeeprom(0,0,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_STATIC_LIGHT); + break; + } + + return state; +} + +void led_set_user(uint8_t usb_led) { + if (usb_led & (1<<USB_LED_CAPS_LOCK)) { + rgblight_mode_noeeprom(RGBLIGHT_MODE_ALTERNATING); + } else { + layer_state_set_user(layer_state); + } +} + +void myrgb_toggle(void) { + if (rgbon) { + rgblight_disable_noeeprom(); + rgbon = false; + } else { + rgblight_enable_noeeprom(); + layer_state_set_user(layer_state); + led_set_user(host_keyboard_leds()); + rgbon = true; + } +} + +bool process_record_user(uint16_t keycode, keyrecord_t *record) { + switch(keycode) { + case MYRGB_TG: + if (record->event.pressed) { + myrgb_toggle(); + } + return false; + default: + return true; + } +} + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + + [_DEFLT] = LAYOUT( \ + KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_DEL, \ + KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_ENT, \ + KC_BSPC, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, \ + KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, \ + MO(_FN), KC_LCTL, KC_LGUI, KC_LALT, MO(_LOWER),KC_SPC,KC_SPC,MO(_RAISE),KC_LEFT, KC_DOWN, KC_UP, KC_RGHT \ + ), + + + [_RAISE] = LAYOUT( \ + KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, LCTL(LGUI(KC_LEFT)), LGUI(KC_L), LCTL(LGUI(KC_RGHT)), _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, KC_MPRV, KC_MNXT, KC_CAPS, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, KC_MUTE, KC_VOLD, KC_VOLU, KC_MPLY \ + ), + + + [_LOWER] = LAYOUT( \ + KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, \ + _______, KC_GRV, _______, _______, _______, _______, _______, _______, KC_MINS, KC_EQL, KC_BSLS, _______, \ + _______, _______, _______, KC_LBRC, KC_RBRC, S(KC_9),S(KC_0),S(KC_LBRC),S(KC_RBRC),_______,_______,_______, \ + _______, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______ \ + ), + + + [_FN] = LAYOUT( \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, RESET, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, MYRGB_TG \ + ) +}; diff --git a/keyboards/jj50/keymaps/abstractkb/readme.md b/keyboards/jj50/keymaps/abstractkb/readme.md new file mode 100644 index 0000000000..7ff7e0268a --- /dev/null +++ b/keyboards/jj50/keymaps/abstractkb/readme.md @@ -0,0 +1,6 @@ +# My personal layout for the JJ50 + +It is an approximation of a 60% layout with the alternate layers set up for programming, +with brackets and parenthesis on the homerow +Other things I changed were swapping around delete, backspace, and capslock around +It also uses layer based rgb underglow, and the underglow flashes when capslock is enabled
\ No newline at end of file diff --git a/keyboards/keebio/bfo9000/keymaps/abstractkb/config.h b/keyboards/keebio/bfo9000/keymaps/abstractkb/config.h new file mode 100644 index 0000000000..67c64fd8e8 --- /dev/null +++ b/keyboards/keebio/bfo9000/keymaps/abstractkb/config.h @@ -0,0 +1,37 @@ +/* +This is the c configuration file for the keymap + +Copyright 2012 Jun Wako <wakojun@gmail.com> +Copyright 2015 Jack Humbert +(Modified) Copyright 2019 AbstractKB + +This program is free software: you can redistribute it and/or modify +it under the terms of the GNU General Public License as published by +the Free Software Foundation, either version 2 of the License, or +(at your option) any later version. + +This program is distributed in the hope that it will be useful, +but WITHOUT ANY WARRANTY; without even the implied warranty of +MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the +GNU General Public License for more details. + +You should have received a copy of the GNU General Public License +along with this program. If not, see <http://www.gnu.org/licenses/>. +*/ + +#pragma once + +//my block------------ +#define RGBLIGHT_ANIMATIONS +#define RGBLIGHT_SLEEP + +/* Use I2C or Serial, not both */ + +#define USE_SERIAL +// #define USE_I2C + +/* Select hand configuration */ + +#define MASTER_LEFT +// #define MASTER_RIGHT +// #define EE_HANDS diff --git a/keyboards/keebio/bfo9000/keymaps/abstractkb/keymap.c b/keyboards/keebio/bfo9000/keymaps/abstractkb/keymap.c new file mode 100644 index 0000000000..a8348ecfb5 --- /dev/null +++ b/keyboards/keebio/bfo9000/keymaps/abstractkb/keymap.c @@ -0,0 +1,92 @@ +#include QMK_KEYBOARD_H + +enum layers { + _BASE, + _LIST +}; + +enum my_keycodes { + MYRGB_TG = SAFE_RANGE +}; + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + +[_BASE] = LAYOUT( \ + KC_MPLY, KC_PSLS, KC_PAST, KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, MYRGB_TG, KC_PGUP, \ + KC_MUTE, KC_PPLS, KC_PMNS, KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_DEL, KC_PGDN, \ + KC_P7, KC_P8, KC_P9, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_HOME, \ + KC_P4, KC_P5, KC_P6, KC_BSPACE, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_CAPS, KC_PSCR, \ + KC_P1, KC_P2, KC_P3, KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, XXXXXXX, KC_INS, \ + KC_P0, KC_PDOT, KC_PENT, TO(_LIST), KC_LCTL, KC_LGUI, KC_LALT, XXXXXXX, KC_SPC, KC_SPC, XXXXXXX, KC_RALT, KC_RGUI, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT, XXXXXXX \ +), + +[_LIST] = LAYOUT( \ + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, + _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, + _______, _______, _______, TO(_BASE), _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______ +) + +}; + +bool rgbinit = true; +bool rgbon = true; + +const uint8_t RGBLED_RAINBOW_SWIRL_INTERVALS[] PROGMEM = {1,5,5}; //only using the first one + +void matrix_post_init_user(void) { + rgblight_enable_noeeprom(); + led_set_user(host_keyboard_leds()); +} + +uint32_t layer_state_set_user(uint32_t state) { + switch (biton32(state)) { + case _LIST: + rgblight_sethsv_noeeprom(0,255,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_RAINBOW_SWIRL); + break; + default: //_BASE + rgblight_sethsv_noeeprom(0,0,255); + rgblight_mode_noeeprom(RGBLIGHT_MODE_STATIC_LIGHT); + break; + } + + return state; +} + +void led_set_user(uint8_t usb_led) { + if (usb_led & (1<<USB_LED_CAPS_LOCK)) { + rgblight_mode_noeeprom(RGBLIGHT_MODE_ALTERNATING); + } else { + layer_state_set_user(layer_state); + } +} + +void myrgb_toggle(void) { + if (rgbon) { + rgblight_disable_noeeprom(); + rgbon = false; + } else { + rgblight_enable_noeeprom(); + layer_state_set_user(layer_state); + led_set_user(host_keyboard_leds()); + rgbon = true; + } +} + +bool process_record_user(uint16_t keycode, keyrecord_t *record) { + switch(keycode) { + case MYRGB_TG: + if (record->event.pressed) { + myrgb_toggle(); + } + return false; + default: + return true; + } +} + + + diff --git a/keyboards/keebio/bfo9000/keymaps/abstractkb/readme.md b/keyboards/keebio/bfo9000/keymaps/abstractkb/readme.md new file mode 100644 index 0000000000..3ba56571fe --- /dev/null +++ b/keyboards/keebio/bfo9000/keymaps/abstractkb/readme.md @@ -0,0 +1,5 @@ +# My personal layout for the BFO-9000 + +It is an approximation of a 96% layout with a left-hand number pad +Other things I changed were swapping around delete, backspace, and capslock +It also uses layer based rgb underglow, and the underglow flashes when capslock is enabled
\ No newline at end of file diff --git a/keyboards/keebio/bfo9000/keymaps/abstractkb/rules.mk b/keyboards/keebio/bfo9000/keymaps/abstractkb/rules.mk new file mode 100644 index 0000000000..7ad666d1a3 --- /dev/null +++ b/keyboards/keebio/bfo9000/keymaps/abstractkb/rules.mk @@ -0,0 +1 @@ +RGBLIGHT_ENABLE = yes
\ No newline at end of file diff --git a/keyboards/quantrik/kyuu/config.h b/keyboards/quantrik/kyuu/config.h new file mode 100644 index 0000000000..655314985d --- /dev/null +++ b/keyboards/quantrik/kyuu/config.h @@ -0,0 +1,245 @@ +/* +Copyright 2019 mechmerlin + +This program is free software: you can redistribute it and/or modify +it under the terms of the GNU General Public License as published by +the Free Software Foundation, either version 2 of the License, or +(at your option) any later version. + +This program is distributed in the hope that it will be useful, +but WITHOUT ANY WARRANTY; without even the implied warranty of +MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the +GNU General Public License for more details. + +You should have received a copy of the GNU General Public License +along with this program. If not, see <http://www.gnu.org/licenses/>. +*/ + +#pragma once + +#include "config_common.h" + +/* USB Device descriptor parameter */ +#define VENDOR_ID 0xFEED +#define PRODUCT_ID 0x0000 +#define DEVICE_VER 0x0001 +#define MANUFACTURER Quantrik +#define PRODUCT Kyuu +#define DESCRIPTION A 65% keyboard with blocker + +/* key matrix size */ +#define MATRIX_ROWS 5 +#define MATRIX_COLS 15 + +/* + * Keyboard Matrix Assignments + * + * Change this to how you wired your keyboard + * COLS: AVR pins used for columns, left to right + * ROWS: AVR pins used for rows, top to bottom + * DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode) + * ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode) + * +*/ +#define MATRIX_ROW_PINS { B6, B5, B4, D7, D6 } +#define MATRIX_COL_PINS { F1, F4, F5, F6, F7, C7, C6, F0, B7, D0, D5, D3, D2, D1, B3 } +#define UNUSED_PINS + +/* COL2ROW, ROW2COL*/ +#define DIODE_DIRECTION COL2ROW + +/* + * Split Keyboard specific options, make sure you have 'SPLIT_KEYBOARD = yes' in your rules.mk, and define SOFT_SERIAL_PIN. + */ +// #define SOFT_SERIAL_PIN D0 // or D1, D2, D3, E6 + +// #define BACKLIGHT_PIN B7 +// #define BACKLIGHT_BREATHING +// #define BACKLIGHT_LEVELS 3 + +// #define RGB_DI_PIN E2 +// #ifdef RGB_DI_PIN +// #define RGBLED_NUM 16 +// #define RGBLIGHT_HUE_STEP 8 +// #define RGBLIGHT_SAT_STEP 8 +// #define RGBLIGHT_VAL_STEP 8 +// #define RGBLIGHT_LIMIT_VAL 255 /* The maximum brightness level */ +// #define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */ +// /*== all animations enable ==*/ +// #define RGBLIGHT_ANIMATIONS +// /*== or choose animations ==*/ +// #define RGBLIGHT_EFFECT_BREATHING +// #define RGBLIGHT_EFFECT_RAINBOW_MOOD +// #define RGBLIGHT_EFFECT_RAINBOW_SWIRL +// #define RGBLIGHT_EFFECT_SNAKE +// #define RGBLIGHT_EFFECT_KNIGHT +// #define RGBLIGHT_EFFECT_CHRISTMAS +// #define RGBLIGHT_EFFECT_STATIC_GRADIENT +// #define RGBLIGHT_EFFECT_RGB_TEST +// #define RGBLIGHT_EFFECT_ALTERNATING +// #endif + +/* Debounce reduces chatter (unintended double-presses) - set 0 if debouncing is not needed */ +#define DEBOUNCING_DELAY 5 + +/* define if matrix has ghost (lacks anti-ghosting diodes) */ +//#define MATRIX_HAS_GHOST + +/* number of backlight levels */ + +/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */ +#define LOCKING_SUPPORT_ENABLE +/* Locking resynchronize hack */ +#define LOCKING_RESYNC_ENABLE + +/* If defined, GRAVE_ESC will always act as ESC when CTRL is held. + * This is userful for the Windows task manager shortcut (ctrl+shift+esc). + */ +// #define GRAVE_ESC_CTRL_OVERRIDE + +/* + * Force NKRO + * + * Force NKRO (nKey Rollover) to be enabled by default, regardless of the saved + * state in the bootmagic EEPROM settings. (Note that NKRO must be enabled in the + * makefile for this to work.) + * + * If forced on, NKRO can be disabled via magic key (default = LShift+RShift+N) + * until the next keyboard reset. + * + * NKRO may prevent your keystrokes from being detected in the BIOS, but it is + * fully operational during normal computer usage. + * + * For a less heavy-handed approach, enable NKRO via magic key (LShift+RShift+N) + * or via bootmagic (hold SPACE+N while plugging in the keyboard). Once set by + * bootmagic, NKRO mode will always be enabled until it is toggled again during a + * power-up. + * + */ +//#define FORCE_NKRO + +/* + * Magic Key Options + * + * Magic keys are hotkey commands that allow control over firmware functions of + * the keyboard. They are best used in combination with the HID Listen program, + * found here: https://www.pjrc.com/teensy/hid_listen.html + * + * The options below allow the magic key functionality to be changed. This is + * useful if your keyboard/keypad is missing keys and you want magic key support. + * + */ + +/* key combination for magic key command */ +/* defined by default; to change, uncomment and set to the combination you want */ +// #define IS_COMMAND() (get_mods() == (MOD_BIT(KC_LSHIFT) | MOD_BIT(KC_RSHIFT))) + +/* control how magic key switches layers */ +//#define MAGIC_KEY_SWITCH_LAYER_WITH_FKEYS true +//#define MAGIC_KEY_SWITCH_LAYER_WITH_NKEYS true +//#define MAGIC_KEY_SWITCH_LAYER_WITH_CUSTOM false + +/* override magic key keymap */ +//#define MAGIC_KEY_SWITCH_LAYER_WITH_FKEYS +//#define MAGIC_KEY_SWITCH_LAYER_WITH_NKEYS +//#define MAGIC_KEY_SWITCH_LAYER_WITH_CUSTOM +//#define MAGIC_KEY_HELP H +//#define MAGIC_KEY_HELP_ALT SLASH +//#define MAGIC_KEY_DEBUG D +//#define MAGIC_KEY_DEBUG_MATRIX X +//#define MAGIC_KEY_DEBUG_KBD K +//#define MAGIC_KEY_DEBUG_MOUSE M +//#define MAGIC_KEY_VERSION V +//#define MAGIC_KEY_STATUS S +//#define MAGIC_KEY_CONSOLE C +//#define MAGIC_KEY_LAYER0 0 +//#define MAGIC_KEY_LAYER0_ALT GRAVE +//#define MAGIC_KEY_LAYER1 1 +//#define MAGIC_KEY_LAYER2 2 +//#define MAGIC_KEY_LAYER3 3 +//#define MAGIC_KEY_LAYER4 4 +//#define MAGIC_KEY_LAYER5 5 +//#define MAGIC_KEY_LAYER6 6 +//#define MAGIC_KEY_LAYER7 7 +//#define MAGIC_KEY_LAYER8 8 +//#define MAGIC_KEY_LAYER9 9 +//#define MAGIC_KEY_BOOTLOADER B +//#define MAGIC_KEY_BOOTLOADER_ALT ESC +//#define MAGIC_KEY_LOCK CAPS +//#define MAGIC_KEY_EEPROM E +//#define MAGIC_KEY_EEPROM_CLEAR BSPACE +//#define MAGIC_KEY_NKRO N +//#define MAGIC_KEY_SLEEP_LED Z + +/* + * Feature disable options + * These options are also useful to firmware size reduction. + */ + +/* disable debug print */ +//#define NO_DEBUG + +/* disable print */ +//#define NO_PRINT + +/* disable action features */ +//#define NO_ACTION_LAYER +//#define NO_ACTION_TAPPING +//#define NO_ACTION_ONESHOT +//#define NO_ACTION_MACRO +//#define NO_ACTION_FUNCTION + +/* + * MIDI options + */ + +/* Prevent use of disabled MIDI features in the keymap */ +//#define MIDI_ENABLE_STRICT 1 + +/* enable basic MIDI features: + - MIDI notes can be sent when in Music mode is on +*/ +//#define MIDI_BASIC + +/* enable advanced MIDI features: + - MIDI notes can be added to the keymap + - Octave shift and transpose + - Virtual sustain, portamento, and modulation wheel + - etc. +*/ +//#define MIDI_ADVANCED + +/* override number of MIDI tone keycodes (each octave adds 12 keycodes and allocates 12 bytes) */ +//#define MIDI_TONE_KEYCODE_OCTAVES 1 + +/* + * HD44780 LCD Display Configuration + */ +/* +#define LCD_LINES 2 //< number of visible lines of the display +#define LCD_DISP_LENGTH 16 //< visibles characters per line of the display + +#define LCD_IO_MODE 1 //< 0: memory mapped mode, 1: IO port mode + +#if LCD_IO_MODE +#define LCD_PORT PORTB //< port for the LCD lines +#define LCD_DATA0_PORT LCD_PORT //< port for 4bit data bit 0 +#define LCD_DATA1_PORT LCD_PORT //< port for 4bit data bit 1 +#define LCD_DATA2_PORT LCD_PORT //< port for 4bit data bit 2 +#define LCD_DATA3_PORT LCD_PORT //< port for 4bit data bit 3 +#define LCD_DATA0_PIN 4 //< pin for 4bit data bit 0 +#define LCD_DATA1_PIN 5 //< pin for 4bit data bit 1 +#define LCD_DATA2_PIN 6 //< pin for 4bit data bit 2 +#define LCD_DATA3_PIN 7 //< pin for 4bit data bit 3 +#define LCD_RS_PORT LCD_PORT //< port for RS line +#define LCD_RS_PIN 3 //< pin for RS line +#define LCD_RW_PORT LCD_PORT //< port for RW line +#define LCD_RW_PIN 2 //< pin for RW line +#define LCD_E_PORT LCD_PORT //< port for Enable line +#define LCD_E_PIN 1 //< pin for Enable line +#endif +*/ + +/* Bootmagic Lite key configuration */ +// #define BOOTMAGIC_LITE_ROW 0 +// #define BOOTMAGIC_LITE_COLUMN 0 diff --git a/keyboards/quantrik/kyuu/info.json b/keyboards/quantrik/kyuu/info.json new file mode 100644 index 0000000000..05c1b40b95 --- /dev/null +++ b/keyboards/quantrik/kyuu/info.json @@ -0,0 +1,82 @@ +{ + "keyboard_name": "Kyuu", + "url": "", + "maintainer": "qmk", + "width": 16, + "height": 5, + "layouts": { + "LAYOUT": { + "key_count": 67, + "layout": [ + {"label":"K00 (B6,F1)", "x":0, "y":0}, + {"label":"K01 (B6,F4)", "x":1, "y":0}, + {"label":"K02 (B6,F5)", "x":2, "y":0}, + {"label":"K03 (B6,F6)", "x":3, "y":0}, + {"label":"K04 (B6,F7)", "x":4, "y":0}, + {"label":"K05 (B6,C7)", "x":5, "y":0}, + {"label":"K06 (B6,C6)", "x":6, "y":0}, + {"label":"K07 (B6,F0)", "x":7, "y":0}, + {"label":"K08 (B6,B7)", "x":8, "y":0}, + {"label":"K09 (B6,D0)", "x":9, "y":0}, + {"label":"K0A (B6,D5)", "x":10, "y":0}, + {"label":"K0B (B6,D3)", "x":11, "y":0}, + {"label":"K0C (B6,D2)", "x":12, "y":0}, + {"label":"K0D (B6,D1)", "x":13, "y":0}, + {"label":"K0E (B6,B3)", "x":14, "y":0}, + {"label":"K2E (B4,B3)", "x":15, "y":0}, + {"label":"K10 (B5,F1)", "x":0, "y":1, "w":1.5}, + {"label":"K11 (B5,F4)", "x":1.5, "y":1}, + {"label":"K12 (B5,F5)", "x":2.5, "y":1}, + {"label":"K13 (B5,F6)", "x":3.5, "y":1}, + {"label":"K14 (B5,F7)", "x":4.5, "y":1}, + {"label":"K15 (B5,C7)", "x":5.5, "y":1}, + {"label":"K16 (B5,C6)", "x":6.5, "y":1}, + {"label":"K17 (B5,F0)", "x":7.5, "y":1}, + {"label":"K18 (B5,B7)", "x":8.5, "y":1}, + {"label":"K19 (B5,D0)", "x":9.5, "y":1}, + {"label":"K1A (B5,D5)", "x":10.5, "y":1}, + {"label":"K1B (B5,D3)", "x":11.5, "y":1}, + {"label":"K1C (B5,D2)", "x":12.5, "y":1}, + {"label":"K1D (B5,D1)", "x":13.5, "y":1, "w":1.5}, + {"label":"K1E (B5,B3)", "x":15, "y":1}, + {"label":"K20 (B4,F1)", "x":0, "y":2, "w":1.75}, + {"label":"K21 (B4,F4)", "x":1.75, "y":2}, + {"label":"K22 (B4,F5)", "x":2.75, "y":2}, + {"label":"K23 (B4,F6)", "x":3.75, "y":2}, + {"label":"K24 (B4,F7)", "x":4.75, "y":2}, + {"label":"K25 (B4,C7)", "x":5.75, "y":2}, + {"label":"K26 (B4,C6)", "x":6.75, "y":2}, + {"label":"K27 (B4,F0)", "x":7.75, "y":2}, + {"label":"K28 (B4,B7)", "x":8.75, "y":2}, + {"label":"K29 (B4,D0)", "x":9.75, "y":2}, + {"label":"K2A (B4,D5)", "x":10.75, "y":2}, + {"label":"K2B (B4,D3)", "x":11.75, "y":2}, + {"label":"K2C (B4,D2)", "x":12.75, "y":2, "w":2.25}, + {"label":"K2D (B4,D1)", "x":15, "y":2}, + {"label":"K30 (D7,F1)", "x":0, "y":3, "w":2.25}, + {"label":"K31 (D7,F4)", "x":2.25, "y":3}, + {"label":"K32 (D7,F5)", "x":3.25, "y":3}, + {"label":"K33 (D7,F6)", "x":4.25, "y":3}, + {"label":"K34 (D7,F7)", "x":5.25, "y":3}, + {"label":"K35 (D7,C7)", "x":6.25, "y":3}, + {"label":"K36 (D7,C6)", "x":7.25, "y":3}, + {"label":"K37 (D7,F0)", "x":8.25, "y":3}, + {"label":"K38 (D7,B7)", "x":9.25, "y":3}, + {"label":"K39 (D7,D0)", "x":10.25, "y":3}, + {"label":"K3A (D7,D5)", "x":11.25, "y":3}, + {"label":"K3B (D7,D3)", "x":12.25, "y":3, "w":1.75}, + {"label":"K3C (D7,D2)", "x":14, "y":3}, + {"label":"K3D (D7,D1)", "x":15, "y":3}, + {"label":"K40 (D6,F1)", "x":0, "y":4, "w":1.5}, + {"label":"K41 (D6,F4)", "x":1.5, "y":4}, + {"label":"K42 (D6,F5)", "x":2.5, "y":4, "w":1.5}, + {"label":"K46 (D6,C6)", "x":4, "y":4, "w":7}, + {"label":"K4A (D6,D5)", "x":11, "y":4, "w":1.5}, + {"label":"K4B (D6,D3)", "x":13, "y":4}, + {"label":"K4C (D6,D2)", "x":14, "y":4}, + {"label":"K4D (D6,D1)", "x":15, "y":4} + ] + } + } + } +
\ No newline at end of file diff --git a/keyboards/quantrik/kyuu/keymaps/default/config.h b/keyboards/quantrik/kyuu/keymaps/default/config.h new file mode 100644 index 0000000000..60dd02a9d0 --- /dev/null +++ b/keyboards/quantrik/kyuu/keymaps/default/config.h @@ -0,0 +1,19 @@ +/* Copyright 2019 mechmerlin + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ + +#pragma once + +// place overrides here diff --git a/keyboards/quantrik/kyuu/keymaps/default/keymap.c b/keyboards/quantrik/kyuu/keymaps/default/keymap.c new file mode 100644 index 0000000000..077d7ac5a6 --- /dev/null +++ b/keyboards/quantrik/kyuu/keymaps/default/keymap.c @@ -0,0 +1,74 @@ +/* Copyright 2019 mechmerlin + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include QMK_KEYBOARD_H + +// Defines the keycodes used by our macros in process_record_user +enum custom_keycodes { + QMKBEST = SAFE_RANGE, + QMKURL +}; + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + [0] = LAYOUT( \ + KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSLS, KC_GRV, KC_HOME, \ + KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSPC, KC_PGUP, \ + KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_PGDN, \ + KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_LSFT, KC_UP, KC_END, \ + KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, MO(1), KC_LEFT, KC_DOWN, KC_RGHT \ + ), + [1] = LAYOUT( \ + KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, \ + KC_TRNS, QMKBEST, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, RESET, KC_TRNS, \ + KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, \ + KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, \ + KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS \ + ), + +}; + +bool process_record_user(uint16_t keycode, keyrecord_t *record) { + switch (keycode) { + case QMKBEST: + if (record->event.pressed) { + // when keycode QMKBEST is pressed + SEND_STRING("QMK is the best thing ever!"); + } else { + // when keycode QMKBEST is released + } + break; + case QMKURL: + if (record->event.pressed) { + // when keycode QMKURL is pressed + SEND_STRING("https://qmk.fm/" SS_TAP(X_ENTER)); + } else { + // when keycode QMKURL is released + } + break; + } + return true; +} + +void matrix_init_user(void) { + +} + +void matrix_scan_user(void) { + +} + +void led_set_user(uint8_t usb_led) { + +} diff --git a/keyboards/quantrik/kyuu/keymaps/default/readme.md b/keyboards/quantrik/kyuu/keymaps/default/readme.md new file mode 100644 index 0000000000..f87ce36e38 --- /dev/null +++ b/keyboards/quantrik/kyuu/keymaps/default/readme.md @@ -0,0 +1 @@ +# The default keymap for Kyuu diff --git a/keyboards/quantrik/kyuu/kyuu.c b/keyboards/quantrik/kyuu/kyuu.c new file mode 100644 index 0000000000..e9a72a5749 --- /dev/null +++ b/keyboards/quantrik/kyuu/kyuu.c @@ -0,0 +1,43 @@ +/* Copyright 2019 mechmerlin + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#include "kyuu.h" + +void matrix_init_kb(void) { + // put your keyboard start-up code here + // runs once when the firmware starts up + + matrix_init_user(); +} + +void matrix_scan_kb(void) { + // put your looping keyboard code here + // runs every cycle (a lot) + + matrix_scan_user(); +} + +bool process_record_kb(uint16_t keycode, keyrecord_t *record) { + // put your per-action keyboard code here + // runs for every action, just before processing by the firmware + + return process_record_user(keycode, record); +} + +void led_set_kb(uint8_t usb_led) { + // put your keyboard LED indicator (ex: Caps Lock LED) toggling code here + + led_set_user(usb_led); +} diff --git a/keyboards/quantrik/kyuu/kyuu.h b/keyboards/quantrik/kyuu/kyuu.h new file mode 100644 index 0000000000..82c024f2e7 --- /dev/null +++ b/keyboards/quantrik/kyuu/kyuu.h @@ -0,0 +1,42 @@ +/* Copyright 2019 mechmerlin + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ +#pragma once + +#include "quantum.h" + +/* This a shortcut to help you visually see your layout. + * + * The first section contains all of the arguments representing the physical + * layout of the board and position of the keys. + * + * The second converts the arguments into a two-dimensional array which + * represents the switch matrix. + */ +#define LAYOUT( \ + k00, k01, k02, k03, k04, k05, k06, k07, k08, k09, k0A, k0B, k0C, k0D, k0E, k2E, \ + k10, k11, k12, k13, k14, k15, k16, k17, k18, k19, k1A, k1B, k1C, k1D, k1E, \ + k20, k21, k22, k23, k24, k25, k26, k27, k28, k29, k2A, k2B, k2C, \ + k30, k31, k32, k33, k34, k35, k36, k37, k38, k39, k3A, k3B, k3C, k3D, \ + k40, k41, k42, k46, k47, k4A, k4B, k4C, k4D \ +) \ +{ \ + { k00, k01, k02, k03, k04, k05, k06, k07, k08, k09, k0A, k0B, k0C, k0D, k0E }, \ + { k10, k11, k12, k13, k14, k15, k16, k17, k18, k19, k1A, k1B, k1C, k1D, k1E }, \ + { k20, k21, k22, k23, k24, k25, k26, k27, k28, k29, k2A, k2B, k2C, KC_NO, k2E }, \ + { k30, k31, k32, k33, k34, k35, k36, k37, k38, k39, k3A, k3B, k3C, k3D, KC_NO }, \ + { k40, k41, k42, KC_NO, KC_NO, KC_NO, k46, k47, KC_NO, KC_NO, k4A, k4B, k4C, k4D, KC_NO }, \ +} + diff --git a/keyboards/quantrik/kyuu/readme.md b/keyboards/quantrik/kyuu/readme.md new file mode 100644 index 0000000000..2b33140980 --- /dev/null +++ b/keyboards/quantrik/kyuu/readme.md @@ -0,0 +1,13 @@ +# kyuu + +Kyuu is a 65% keyboard inspired by the TGR 910's top right blocker. + +Keyboard Maintainer: [MechMerlin](https://github.com/mechmerlin) +Hardware Supported: Quantrik 65% PCB +Hardware Availability: [Geekhack Group Buy](https://geekhack.org/index.php?topic=97810.0), [quantrik.com](https://www.quantrik.com/collections/frontpage/products/kyuu-keyboard) + +Make example for this keyboard (after setting up your build environment): + + make quantrik/kyuu:default + +See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs). diff --git a/keyboards/quantrik/kyuu/rules.mk b/keyboards/quantrik/kyuu/rules.mk new file mode 100644 index 0000000000..3229957d9c --- /dev/null +++ b/keyboards/quantrik/kyuu/rules.mk @@ -0,0 +1,81 @@ +# MCU name +#MCU = at90usb1286 +MCU = atmega32u4 + +# Processor frequency. +# This will define a symbol, F_CPU, in all source code files equal to the +# processor frequency in Hz. You can then use this symbol in your source code to +# calculate timings. Do NOT tack on a 'UL' at the end, this will be done +# automatically to create a 32-bit value in your source code. +# +# This will be an integer division of F_USB below, as it is sourced by +# F_USB after it has run through any CPU prescalers. Note that this value +# does not *change* the processor frequency - it should merely be updated to +# reflect the processor speed set externally so that the code can use accurate +# software delays. +F_CPU = 16000000 + + +# +# LUFA specific +# +# Target architecture (see library "Board Types" documentation). +ARCH = AVR8 + +# Input clock frequency. +# This will define a symbol, F_USB, in all source code files equal to the +# input clock frequency (before any prescaling is performed) in Hz. This value may +# differ from F_CPU if prescaling is used on the latter, and is required as the +# raw input clock is fed directly to the PLL sections of the AVR for high speed +# clock generation for the USB and other AVR subsections. Do NOT tack on a 'UL' +# at the end, this will be done automatically to create a 32-bit value in your +# source code. +# +# If no clock division is performed on the input clock inside the AVR (via the +# CPU clock adjust registers or the clock division fuses), this will be equal to F_CPU. +F_USB = $(F_CPU) + +# Interrupt driven control endpoint task(+60) +OPT_DEFS += -DINTERRUPT_CONTROL_ENDPOINT + + +# Bootloader selection +# Teensy halfkay +# Pro Micro caterina +# Atmel DFU atmel-dfu +# LUFA DFU lufa-dfu +# QMK DFU qmk-dfu +# atmega32a bootloadHID +BOOTLOADER = atmel-dfu + + +# If you don't know the bootloader type, then you can specify the +# Boot Section Size in *bytes* by uncommenting out the OPT_DEFS line +# Teensy halfKay 512 +# Teensy++ halfKay 1024 +# Atmel DFU loader 4096 +# LUFA bootloader 4096 +# USBaspLoader 2048 +# OPT_DEFS += -DBOOTLOADER_SIZE=4096 + + +# Build Options +# change yes to no to disable +# +BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = yes # Mouse keys(+4700) +EXTRAKEY_ENABLE = yes # Audio control and System control(+450) +CONSOLE_ENABLE = yes # Console for debug(+400) +COMMAND_ENABLE = yes # Commands for debug and configuration +# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend +# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work +NKRO_ENABLE = no # USB Nkey Rollover +BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality on B7 by default +RGBLIGHT_ENABLE = no # Enable keyboard RGB underglow +MIDI_ENABLE = no # MIDI support (+2400 to 4200, depending on config) +UNICODE_ENABLE = no # Unicode +BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID +AUDIO_ENABLE = no # Audio output on port C6 +FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches +HD44780_ENABLE = no # Enable support for HD44780 based LCDs (+400) diff --git a/keyboards/romac/config.h b/keyboards/romac/config.h new file mode 100644 index 0000000000..b92785fd52 --- /dev/null +++ b/keyboards/romac/config.h @@ -0,0 +1,40 @@ +#pragma once + +#include "config_common.h" + +/* USB Device descriptor parameter */ +#define VENDOR_ID 0xFEED +#define PRODUCT_ID 0x6060 +#define DEVICE_VER 0x0001 +#define MANUFACTURER TheRoyalSweatshirt +#define PRODUCT romac +#define DESCRIPTION A *Plaid inspired twelve-key macropad +/* key matrix size */ +#define MATRIX_ROWS 4 +#define MATRIX_COLS 3 + +/* key matrix pins */ +#define MATRIX_ROW_PINS { D4, C6, D7, E6 } +#define MATRIX_COL_PINS { F7, B1, B3 } +#define UNUSED_PINS + +/* COL2ROW or ROW2COL */ +#define DIODE_DIRECTION COL2ROW + +/* number of backlight levels */ + +#define BACKLIGHT_LEVELS 0 + +/* Set 0 if debouncing isn't needed */ +#define DEBOUNCING_DELAY 5 + +/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */ +#define LOCKING_SUPPORT_ENABLE + +/* Locking resynchronize hack */ +#define LOCKING_RESYNC_ENABLE + +#define RGBLED_NUM 0 +#define RGBLIGHT_HUE_STEP 8 +#define RGBLIGHT_SAT_STEP 8 +#define RGBLIGHT_VAL_STEP 8 diff --git a/keyboards/romac/info.json b/keyboards/romac/info.json new file mode 100644 index 0000000000..305785991e --- /dev/null +++ b/keyboards/romac/info.json @@ -0,0 +1,13 @@ +{ + "keyboard_name": "RoMac", + "url": "", + "maintainer": "TheRoyalSweatshirt", + "width": 3, + "height": 4, + "layouts": { + "LAYOUT": { + "key_count": 12, + "layout": [{"x":0, "y":0}, {"x":1, "y":0}, {"x":2, "y":0}, {"x":0, "y":1}, {"x":1, "y":1}, {"x":2, "y":1}, {"x":0, "y":2}, {"x":1, "y":2}, {"x":2, "y":2}, {"x":0, "y":3}, {"x":1, "y":3}, {"x":2, "y":3}] + } + } +} diff --git a/keyboards/romac/keymaps/default/keymap.c b/keyboards/romac/keymaps/default/keymap.c new file mode 100644 index 0000000000..35636f36b3 --- /dev/null +++ b/keyboards/romac/keymaps/default/keymap.c @@ -0,0 +1,37 @@ +/* Copyright 2018 Jack Humbert + * + * This program is free software: you can redistribute it and/or modify + * it under the terms of the GNU General Public License as published by + * the Free Software Foundation, either version 2 of the License, or + * (at your option) any later version. + * + * This program is distributed in the hope that it will be useful, + * but WITHOUT ANY WARRANTY; without even the implied warranty of + * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the + * GNU General Public License for more details. + * + * You should have received a copy of the GNU General Public License + * along with this program. If not, see <http://www.gnu.org/licenses/>. + */ + +#include QMK_KEYBOARD_H + +#define _BASE 0 +#define _FN1 1 + +const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { + + [_BASE] = LAYOUT( + KC_7, KC_8, KC_9, \ + KC_4, KC_5, KC_6, \ + KC_1, KC_2, KC_3, \ + MO(_FN1), KC_0, KC_ENT \ + ), + + [_FN1] = LAYOUT( + KC_TRNS, KC_HOME, KC_PGUP, \ + KC_TRNS, KC_END, KC_PGDN, \ + KC_TRNS, KC_TRNS, KC_TRNS, \ + KC_TRNS, KC_TRNS, KC_DOT \ + ) +}; diff --git a/keyboards/romac/readme.md b/keyboards/romac/readme.md new file mode 100644 index 0000000000..98ed21502a --- /dev/null +++ b/keyboards/romac/readme.md @@ -0,0 +1,16 @@ + +# RoMac + + + +A “Plaid” Inspired 12-Key (3x4) Macropad. + +- Keyboard Maintainer: [Garret G.](https://github.com/TheRoyalSweatshirt) +- Hardware Supported: RoMac rev.1, rev.2, Pro Micro, Elite-C, Proton C, BlueMicro. +- Hardware Availability: Through GB or Direct Message (If extra stock is available). + +Make example for this keyboard (after setting up your build environment): + + make romac:default + +See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs). diff --git a/keyboards/romac/romac.c b/keyboards/romac/romac.c new file mode 100644 index 0000000000..24f4c16333 --- /dev/null +++ b/keyboards/romac/romac.c @@ -0,0 +1 @@ +#include "romac.h" diff --git a/keyboards/romac/romac.h b/keyboards/romac/romac.h new file mode 100644 index 0000000000..e6fb9694d2 --- /dev/null +++ b/keyboards/romac/romac.h @@ -0,0 +1,16 @@ +#pragma once + +#include "quantum.h" + +#define LAYOUT( \ + K00, K01, K02, \ + K10, K11, K12, \ + K20, K21, K22, \ + K30, K31, K32 \ +) \ +{ \ + { K00, K01, K02 }, \ + { K10, K11, K12 }, \ + { K20, K21, K22 }, \ + { K30, K31, K32 } \ +} diff --git a/keyboards/romac/rules.mk b/keyboards/romac/rules.mk new file mode 100644 index 0000000000..b500f1b885 --- /dev/null +++ b/keyboards/romac/rules.mk @@ -0,0 +1,57 @@ +# MCU name +MCU = atmega32u4 + +# Processor frequency. +# This will define a symbol, F_CPU, in all source code files equal to the +# processor frequency in Hz. You can then use this symbol in your source code to +# calculate timings. Do NOT tack on a 'UL' at the end, this will be done +# automatically to create a 32-bit value in your source code. +# +# This will be an integer division of F_USB below, as it is sourced by +# F_USB after it has run through any CPU prescalers. Note that this value +# does not *change* the processor frequency - it should merely be updated to +# reflect the processor speed set externally so that the code can use accurate +# software delays. +F_CPU = 16000000 + +# +# LUFA specific +# +# Target architecture (see library "Board Types" documentation). +ARCH = AVR8 + +# Input clock frequency. +# This will define a symbol, F_USB, in all source code files equal to the +# input clock frequency (before any prescaling is performed) in Hz. This value may +# differ from F_CPU if prescaling is used on the latter, and is required as the +# raw input clock is fed directly to the PLL sections of the AVR for high speed +# clock generation for the USB and other AVR subsections. Do NOT tack on a 'UL' +# at the end, this will be done automatically to create a 32-bit value in your +# source code. +# +# If no clock division is performed on the input clock inside the AVR (via the +# CPU clock adjust registers or the clock division fuses), this will be equal to F_CPU. +F_USB = $(F_CPU) + +# Interrupt driven control endpoint task(+60) +OPT_DEFS += -DINTERRUPT_CONTROL_ENDPOINT + + +# Boot Section Size in *bytes* +OPT_DEFS += -DBOOTLOADER_SIZE=4096 + + +# Build Options +# comment out to disable the options. +# +BOOTMAGIC_ENABLE = full # Virtual DIP switch configuration(+1000) +MOUSEKEY_ENABLE = yes # Mouse keys(+4700) +EXTRAKEY_ENABLE = yes # Audio control and System control(+450) +CONSOLE_ENABLE = no # Console for debug(+400) +COMMAND_ENABLE = no # Commands for debug and configuration +SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend +NKRO_ENABLE = yes # USB Nkey Rollover - if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work +BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality +AUDIO_ENABLE = no +RGBLIGHT_ENABLE = no + diff --git a/keyboards/xd75/keymaps/davidrambo/keymap.c b/keyboards/xd75/keymaps/davidrambo/keymap.c index a630affe07..7be197a81c 100644 --- a/keyboards/xd75/keymaps/davidrambo/keymap.c +++ b/keyboards/xd75/keymaps/davidrambo/keymap.c @@ -2,14 +2,14 @@ //aliases for clarity in layering #define A_BSPC LALT(KC_BSPC) // delete whole word in Mac -#define C_BSPS LCTL(KC_BSPC) // delete whole word in PC +#define C_BSPC LCTL(KC_BSPC) // delete whole word in PC #define A_LEFT LALT(KC_LEFT) #define A_RGHT LALT(KC_RGHT) #define C_RGHT LCTL(KC_RGHT) #define C_LEFT LCTL(KC_LEFT) #define SftEnt SFT_T(KC_ENT) -#define GBSPC LGUI_T(KC_BSPC) -#define CBSPC LCTL_T(KC_BSPC) +#define BS_GUI LGUI_T(KC_BSPC) +#define BS_CTL LCTL_T(KC_BSPC) // internet browser tab shortcuts and window/application swapping for Mac and Win #define GSL LGUI(S(KC_LEFT)) // back one tab in Safari @@ -62,7 +62,7 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { KC_GESC, KC_Q, KC_W, KC_F, KC_P, KC_G, KC_LBRC, KC_BSLS, KC_RBRC, KC_J, KC_L, KC_U, KC_Y, KC_SCLN, KC_BSPC, NAV , KC_A, KC_R, KC_S, KC_T, KC_D, KC_PGUP, KC_ESC , KC_ENT , KC_H, KC_N, KC_E, KC_I, KC_O, KC_QUOT, SftLck , KC_Z, KC_X, KC_C, KC_V, KC_B, KC_PGDN, KC_UP , KC_ENT , KC_K, KC_M, KC_COMM, KC_DOT, KC_SLSH, SftEnt , - KC_DEL , KC_LGUI, KC_LCTL, KC_LALT, KC_LGUI, GBSPC, KC_LEFT, KC_DOWN, KC_RGHT, KC_SPC, MO(3) , KC_RGUI, KC_RALT, KC_RCTL, BL_STEP + KC_DEL , KC_LGUI, KC_LCTL, KC_LALT, KC_LGUI, BS_GUI, KC_LEFT, KC_DOWN, KC_RGHT, KC_SPC, MO(3) , KC_RGUI, KC_RALT, KC_RCTL, BL_STEP ), // Windows Layer: essentially swaps Control and GUI @@ -72,7 +72,7 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, NAVPC , _______, _______, _______, _______, _______, _______, KC_MPLY, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, - _______, KC_LCTL, KC_LGUI, _______, KC_LCTL, _______, _______, _______, _______, _______, _______, KC_RCTL, KC_RALT, KC_RGUI, _______ + _______, KC_LCTL, KC_LGUI, _______, KC_LCTL, BS_CTL , _______, _______, _______, _______, _______, KC_RCTL, KC_RALT, KC_RGUI, _______ ), [_GAME] = LAYOUT_ortho_5x15( /* Gaming Layer */ @@ -118,7 +118,7 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, C_TAB , C_LEFT, KC_UP, C_RGHT , KC_DEL , _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, CTLPGUP, KC_LEFT, KC_DOWN, KC_RGHT, CTLPGDN, _______, - _______, _______, _______, _______, _______, _______, _______, _______, _______, A_TAB , CBSPC , KC_HOME, KC_END , _______, _______, + _______, _______, _______, _______, _______, _______, _______, _______, _______, A_TAB , C_BSPC , KC_HOME, KC_END , _______, _______, RESET , _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______ ) }; diff --git a/keyboards/zeal60/config.h b/keyboards/zeal60/config.h index e39b06cf6b..94cc9989b7 100644 --- a/keyboards/zeal60/config.h +++ b/keyboards/zeal60/config.h @@ -100,6 +100,11 @@ #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_3 0b0011000000000001 #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_4 0b0011110000000111 +#define RGB_BACKLIGHT_CAPS_LOCK_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_1_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_2_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_3_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } + #define DYNAMIC_KEYMAP_LAYER_COUNT 4 // EEPROM usage diff --git a/keyboards/zeal60/rgb_backlight.c b/keyboards/zeal60/rgb_backlight.c index a965a13bbd..8d7a7fe8d1 100644 --- a/keyboards/zeal60/rgb_backlight.c +++ b/keyboards/zeal60/rgb_backlight.c @@ -74,10 +74,10 @@ backlight_config g_config = { .effect_speed = 0, .color_1 = { .h = 0, .s = 255 }, .color_2 = { .h = 127, .s = 255 }, - .caps_lock_indicator = { .color = { .h = 0, .s = 0 }, .index = 255 }, - .layer_1_indicator = { .color = { .h = 0, .s = 0 }, .index = 255 }, - .layer_2_indicator = { .color = { .h = 0, .s = 0 }, .index = 255 }, - .layer_3_indicator = { .color = { .h = 0, .s = 0 }, .index = 255 }, + .caps_lock_indicator = RGB_BACKLIGHT_CAPS_LOCK_INDICATOR, + .layer_1_indicator = RGB_BACKLIGHT_LAYER_1_INDICATOR, + .layer_2_indicator = RGB_BACKLIGHT_LAYER_2_INDICATOR, + .layer_3_indicator = RGB_BACKLIGHT_LAYER_3_INDICATOR, .alphas_mods = { RGB_BACKLIGHT_ALPHAS_MODS_ROW_0, RGB_BACKLIGHT_ALPHAS_MODS_ROW_1, diff --git a/keyboards/zeal65/config.h b/keyboards/zeal65/config.h index 7406be865b..896deac8b8 100644 --- a/keyboards/zeal65/config.h +++ b/keyboards/zeal65/config.h @@ -100,6 +100,11 @@ #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_3 0b0111000000000001 #define RGB_BACKLIGHT_ALPHAS_MODS_ROW_4 0b0111110000000011 +#define RGB_BACKLIGHT_CAPS_LOCK_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_1_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_2_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } +#define RGB_BACKLIGHT_LAYER_3_INDICATOR { .color = { .h = 0, .s = 0 }, .index = 255 } + #define DYNAMIC_KEYMAP_LAYER_COUNT 4 // EEPROM usage |